mirror of
https://github.com/Creators-of-Create/Create.git
synced 2024-11-15 23:14:12 +01:00
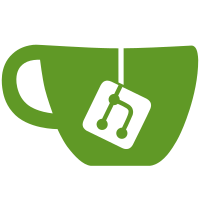
- Added behavioural registry for Spout/Block interaction - Fixed and Refactored TiCon Casting via Spout - Fixed fluid pipes getting stuck trying to waterlog non-solid blocks with fluid handling capabilities - Fixed modlist lookup for non-english locales
42 lines
1007 B
Java
42 lines
1007 B
Java
package com.simibubi.create.compat;
|
|
|
|
import java.util.Optional;
|
|
import java.util.function.Supplier;
|
|
|
|
import com.simibubi.create.foundation.utility.Lang;
|
|
|
|
import net.minecraftforge.fml.ModList;
|
|
|
|
/**
|
|
* For compatibility with and without another mod present, we have to define load conditions of the specific code
|
|
*/
|
|
public enum Mods {
|
|
DYNAMICTREES,
|
|
TCONSTRUCT;
|
|
|
|
/**
|
|
* @return a boolean of whether the mod is loaded or not based on mod id
|
|
*/
|
|
public boolean isLoaded() {
|
|
return ModList.get().isLoaded(asId());
|
|
}
|
|
|
|
/**
|
|
* @return the mod id
|
|
*/
|
|
public String asId() {
|
|
return Lang.asId(name());
|
|
}
|
|
|
|
/**
|
|
* Simple hook to run code if a mod is installed
|
|
* @param toRun will be run only if the mod is loaded
|
|
* @return Optional.empty() if the mod is not loaded, otherwise an Optional of the return value of the given supplier
|
|
*/
|
|
public <T> Optional<T> runIfInstalled(Supplier<Supplier<T>> toRun) {
|
|
if (isLoaded())
|
|
return Optional.of(toRun.get().get());
|
|
return Optional.empty();
|
|
}
|
|
}
|