mirror of
https://github.com/Creators-of-Create/Create.git
synced 2024-11-14 14:34:16 +01:00
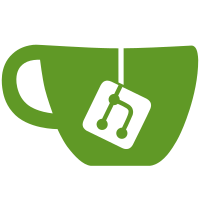
- More cleaning up and major refactoring in Contraption entity implementation (needs testing) - Minecarts can no longer be mounted on seats - Mechanical bearings now "stabilize" rotation of a contraption with an attached sub-contraption
65 lines
2.4 KiB
Java
65 lines
2.4 KiB
Java
package com.simibubi.create;
|
|
|
|
import java.util.HashMap;
|
|
|
|
import javax.annotation.Nullable;
|
|
|
|
import com.simibubi.create.content.contraptions.components.actors.BellMovementBehaviour;
|
|
import com.simibubi.create.content.contraptions.components.actors.CampfireMovementBehaviour;
|
|
import com.simibubi.create.content.contraptions.components.actors.dispenser.DispenserMovementBehaviour;
|
|
import com.simibubi.create.content.contraptions.components.actors.dispenser.DropperMovementBehaviour;
|
|
import com.simibubi.create.content.contraptions.components.structureMovement.MovementBehaviour;
|
|
import com.tterrag.registrate.util.nullness.NonNullConsumer;
|
|
|
|
import net.minecraft.block.Block;
|
|
import net.minecraft.block.BlockState;
|
|
import net.minecraft.block.Blocks;
|
|
import net.minecraft.util.ResourceLocation;
|
|
|
|
public class AllMovementBehaviours {
|
|
private static final HashMap<ResourceLocation, MovementBehaviour> movementBehaviours = new HashMap<>();
|
|
|
|
public static void addMovementBehaviour(ResourceLocation resourceLocation, MovementBehaviour movementBehaviour) {
|
|
if (movementBehaviours.containsKey(resourceLocation))
|
|
Create.logger.warn("Movement behaviour for " + resourceLocation.toString() + " was overridden");
|
|
movementBehaviours.put(resourceLocation, movementBehaviour);
|
|
}
|
|
|
|
public static void addMovementBehaviour(Block block, MovementBehaviour movementBehaviour) {
|
|
addMovementBehaviour(block.getRegistryName(), movementBehaviour);
|
|
}
|
|
|
|
@Nullable
|
|
public static MovementBehaviour of(ResourceLocation resourceLocation) {
|
|
return movementBehaviours.getOrDefault(resourceLocation, null);
|
|
}
|
|
|
|
@Nullable
|
|
public static MovementBehaviour of(Block block) {
|
|
return of(block.getRegistryName());
|
|
}
|
|
|
|
@Nullable
|
|
public static MovementBehaviour of(BlockState state) {
|
|
return of(state.getBlock());
|
|
}
|
|
|
|
public static boolean contains(Block block) {
|
|
return movementBehaviours.containsKey(block.getRegistryName());
|
|
}
|
|
|
|
public static <B extends Block> NonNullConsumer<? super B> addMovementBehaviour(
|
|
MovementBehaviour movementBehaviour) {
|
|
return b -> addMovementBehaviour(b.getRegistryName(), movementBehaviour);
|
|
}
|
|
|
|
static void register() {
|
|
addMovementBehaviour(Blocks.BELL, new BellMovementBehaviour());
|
|
addMovementBehaviour(Blocks.CAMPFIRE, new CampfireMovementBehaviour());
|
|
|
|
DispenserMovementBehaviour.gatherMovedDispenseItemBehaviours();
|
|
addMovementBehaviour(Blocks.DISPENSER, new DispenserMovementBehaviour());
|
|
addMovementBehaviour(Blocks.DROPPER, new DropperMovementBehaviour());
|
|
}
|
|
}
|