mirror of
https://github.com/Creators-of-Create/Create.git
synced 2024-11-14 22:44:07 +01:00
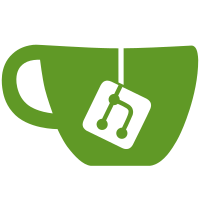
- Fixed Mechanical Press animation de-sync between server and client - The press no longer requires a redstone signal to activate on in-world items - Fixed spouts and basin trying to create potion buckets - The Mechanical bearing can no longer generate force from wind - Added the windmill bearing - Windmill bearings can be configured to rotate counter-clockwise - Added Sail Frames and Sails - Sails can by dyed in-world - Sails negate fall damage - Sails attach themselves to blocks and do not require a sticky surface or chassis - Clockwork bearings can now be configured to switch minute and hour hand aswell as use a 24 hour cycle - Fixed tunnels with windows occluding block faces directly behind them - Fixed item model of the mechanical saw
64 lines
1.3 KiB
Java
64 lines
1.3 KiB
Java
package com.simibubi.create.content;
|
|
|
|
import com.simibubi.create.Create;
|
|
import com.simibubi.create.foundation.item.ItemDescription.Palette;
|
|
|
|
import net.minecraft.block.Block;
|
|
import net.minecraft.item.BlockItem;
|
|
import net.minecraft.item.Item;
|
|
import net.minecraft.item.ItemStack;
|
|
|
|
public enum AllSections {
|
|
|
|
/** Create's kinetic mechanisms */
|
|
KINETICS(Palette.Red),
|
|
|
|
/** Item transport and other Utility */
|
|
LOGISTICS(Palette.Yellow),
|
|
|
|
/** Tools for strucuture movement and replication */
|
|
SCHEMATICS(Palette.Blue),
|
|
|
|
/** Decorative blocks */
|
|
PALETTES(Palette.Green),
|
|
|
|
/** Base materials, ingredients and tools */
|
|
MATERIALS(Palette.Green),
|
|
|
|
/** Helpful gadgets and other shenanigans */
|
|
CURIOSITIES(Palette.Purple),
|
|
|
|
/** Fallback section */
|
|
UNASSIGNED(Palette.Gray)
|
|
|
|
;
|
|
|
|
private Palette tooltipPalette;
|
|
|
|
private AllSections(Palette tooltipPalette) {
|
|
this.tooltipPalette = tooltipPalette;
|
|
}
|
|
|
|
public Palette getTooltipPalette() {
|
|
return tooltipPalette;
|
|
}
|
|
|
|
public static AllSections of(ItemStack stack) {
|
|
Item item = stack.getItem();
|
|
if (item instanceof BlockItem)
|
|
return ofBlock(((BlockItem) item).getBlock());
|
|
return ofItem(item);
|
|
}
|
|
|
|
static AllSections ofItem(Item item) {
|
|
return Create.registrate()
|
|
.getSection(item);
|
|
}
|
|
|
|
static AllSections ofBlock(Block block) {
|
|
return Create.registrate()
|
|
.getSection(block);
|
|
}
|
|
|
|
}
|