mirror of
https://github.com/Jozufozu/Flywheel.git
synced 2024-11-11 13:04:05 +01:00
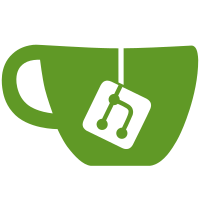
- Moved all block partials used only for rendering out of the registry - Refactored model registry hooks and custom block model handling - Added a safety layer for all tile entity renderers, addresses #76 - Overstressed indicator no longer shows when the kinetic source changes - Fixed framed glass rendering glass textures inbetween blocks - Fixed blockzapper adding itself twice to the item group - Fixed basing not spawning particles properly - Updated Forge - Reworked Schematicannon model
73 lines
2.0 KiB
Java
73 lines
2.0 KiB
Java
package com.simibubi.create;
|
|
|
|
import com.simibubi.create.foundation.block.IHaveNoBlockItem;
|
|
import com.simibubi.create.foundation.item.IAddedByOther;
|
|
|
|
import net.minecraft.block.Block;
|
|
import net.minecraft.client.Minecraft;
|
|
import net.minecraft.client.renderer.ItemRenderer;
|
|
import net.minecraft.client.renderer.model.IBakedModel;
|
|
import net.minecraft.item.ItemGroup;
|
|
import net.minecraft.item.ItemStack;
|
|
import net.minecraft.util.NonNullList;
|
|
import net.minecraftforge.api.distmarker.Dist;
|
|
import net.minecraftforge.api.distmarker.OnlyIn;
|
|
|
|
public final class CreateItemGroup extends ItemGroup {
|
|
|
|
public CreateItemGroup() {
|
|
super(getGroupCountSafe(), Create.ID);
|
|
}
|
|
|
|
@Override
|
|
public ItemStack createIcon() {
|
|
return new ItemStack(AllBlocks.COGWHEEL.get());
|
|
}
|
|
|
|
@Override
|
|
@OnlyIn(Dist.CLIENT)
|
|
public void fill(NonNullList<ItemStack> items) {
|
|
addItems(items, true);
|
|
addBlocks(items);
|
|
addItems(items, false);
|
|
}
|
|
|
|
@OnlyIn(Dist.CLIENT)
|
|
public void addBlocks(NonNullList<ItemStack> items) {
|
|
for (AllBlocks block : AllBlocks.values()) {
|
|
Block def = block.get();
|
|
if (def == null)
|
|
continue;
|
|
if (!block.module.isEnabled())
|
|
continue;
|
|
if (def instanceof IHaveNoBlockItem && !((IHaveNoBlockItem) def).hasBlockItem())
|
|
continue;
|
|
if (def instanceof IAddedByOther)
|
|
continue;
|
|
|
|
def.asItem().fillItemGroup(this, items);
|
|
for (Block alsoRegistered : block.alsoRegistered)
|
|
alsoRegistered.asItem().fillItemGroup(this, items);
|
|
}
|
|
}
|
|
|
|
@OnlyIn(Dist.CLIENT)
|
|
public void addItems(NonNullList<ItemStack> items, boolean specialItems) {
|
|
ItemRenderer itemRenderer = Minecraft.getInstance().getItemRenderer();
|
|
|
|
for (AllItems item : AllItems.values()) {
|
|
if (item.get() == null)
|
|
continue;
|
|
if (!item.module.isEnabled())
|
|
continue;
|
|
IBakedModel model = itemRenderer.getModelWithOverrides(item.asStack());
|
|
if (model.isGui3d() != specialItems)
|
|
continue;
|
|
if (item.get() instanceof IAddedByOther)
|
|
continue;
|
|
|
|
item.get().fillItemGroup(this, items);
|
|
}
|
|
}
|
|
}
|