mirror of
https://github.com/Jozufozu/Flywheel.git
synced 2025-01-10 06:16:07 +01:00
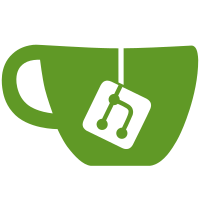
- Program specs are now loaded from json instead of being defined in code and registered manually. - Within the json spec, a program can define a list of states. - A state consists of: - A "when" clause. - A list of strings to be #defined. - A list of extensions to apply at program link time. - Each frame, the first state whose "when" clause returns true will be used. - A when clause consists of: - A state provider defined by a resource location. - A value to match. - When the value returned by the provider matches the value defined in the when clause, the when clause is considered to be 'true'. - There is syntactic sugar for when a provider returns a boolean value. - This system is in its infancy, and there is plenty of room for improvement.
26 lines
647 B
Java
26 lines
647 B
Java
package com.jozufozu.flywheel.util;
|
|
|
|
import java.util.Collections;
|
|
import java.util.List;
|
|
|
|
import com.mojang.datafixers.util.Either;
|
|
import com.mojang.serialization.Codec;
|
|
|
|
public class CodecUtil {
|
|
|
|
/**
|
|
* Creates a list codec that can be parsed from either a single element or a complete list.
|
|
*/
|
|
public static <T> Codec<List<T>> oneOrMore(Codec<T> codec) {
|
|
return Codec.either(codec.listOf(), codec)
|
|
.xmap(
|
|
either -> either.map(l -> l, Collections::singletonList),
|
|
list -> {
|
|
if (list.size() == 1) {
|
|
return Either.right(list.get(0));
|
|
} else {
|
|
return Either.left(list);
|
|
}
|
|
});
|
|
}
|
|
}
|