mirror of
https://github.com/Jozufozu/Flywheel.git
synced 2025-01-10 06:16:07 +01:00
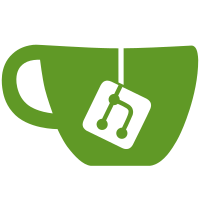
- Removed WIP Items from Creative Tab - Added option to disable tooltips - Moved common config values to synced serverconfig - Numbers on Scrollable Blocks are centered - Motors can be configured to rotate with negative speed - Fixed Processing Recipes Serializer (severe) - Fixed Moving constructs not rendering at a reasonable distance - Mechanical Bearing always solidifies when empty - Fixed some movement incosistencies with translation constructs - Fixed Crushing Wheel Controller not stopping when Wheels do - Fixed Crushing Wheels ejecting entities on world reload - Fixed Movement inconsistencies with Mechanical Belts - Added rotation propagation through large cogwheels connected at a 90 degree angle - Fixed Client code being called server side - Fixed Abstract Method errors regarding Extractors and FrequencyHolders - Added a unit character to Flexpeater display - Fixed additional washing outputs from flying all over the place - Schematicannon now ignores Structure Void blocks - Fixed Schematic hologram not displaying - Added little indicators to the Mechanical Bearing to visualize curent angle - Bumped version
54 lines
1.3 KiB
Java
54 lines
1.3 KiB
Java
package com.simibubi.create.modules;
|
|
|
|
import com.google.gson.JsonObject;
|
|
import com.simibubi.create.Create;
|
|
|
|
import net.minecraft.util.JSONUtils;
|
|
import net.minecraft.util.ResourceLocation;
|
|
import net.minecraftforge.common.crafting.conditions.ICondition;
|
|
import net.minecraftforge.common.crafting.conditions.IConditionSerializer;
|
|
|
|
public class ModuleLoadedCondition implements ICondition {
|
|
|
|
private static final ResourceLocation NAME = new ResourceLocation(Create.ID, "module");
|
|
protected String module;
|
|
|
|
public ModuleLoadedCondition(String module) {
|
|
this.module = module;
|
|
}
|
|
|
|
@Override
|
|
public ResourceLocation getID() {
|
|
return NAME;
|
|
}
|
|
|
|
@Override
|
|
public boolean test() {
|
|
return IModule.isActive(module);
|
|
}
|
|
|
|
@Override
|
|
public String toString() {
|
|
return "module_loaded(\"" + module + "\")";
|
|
}
|
|
|
|
public static class Serializer implements IConditionSerializer<ModuleLoadedCondition> {
|
|
public static final Serializer INSTANCE = new Serializer();
|
|
|
|
@Override
|
|
public void write(JsonObject json, ModuleLoadedCondition value) {
|
|
json.addProperty("module", value.module);
|
|
}
|
|
|
|
@Override
|
|
public ModuleLoadedCondition read(JsonObject json) {
|
|
return new ModuleLoadedCondition(JSONUtils.getString(json, "module"));
|
|
}
|
|
|
|
@Override
|
|
public ResourceLocation getID() {
|
|
return NAME;
|
|
}
|
|
}
|
|
|
|
}
|