mirror of
https://github.com/roddhjav/apparmor.d.git
synced 2024-11-15 07:54:17 +01:00
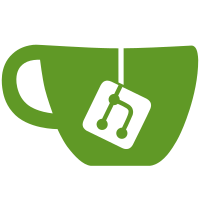
- ABI4 by default, fallback to abi 3. - aa-prebuild cli that can be used by other project shipping profiles. - --file option to cli to only build one dev profile. - add abi version filter to only & exclude directives.
59 lines
1.4 KiB
Go
59 lines
1.4 KiB
Go
// apparmor.d - Full set of apparmor profiles
|
|
// Copyright (C) 2021-2024 Alexandre Pujol <alexandre@pujol.io>
|
|
// SPDX-License-Identifier: GPL-2.0-only
|
|
|
|
package prepare
|
|
|
|
import (
|
|
"github.com/roddhjav/apparmor.d/pkg/paths"
|
|
"github.com/roddhjav/apparmor.d/pkg/prebuild"
|
|
"github.com/roddhjav/apparmor.d/pkg/util"
|
|
)
|
|
|
|
type Synchronise struct {
|
|
prebuild.Base
|
|
Path string
|
|
}
|
|
|
|
func init() {
|
|
RegisterTask(&Synchronise{
|
|
Base: prebuild.Base{
|
|
Keyword: "synchronise",
|
|
Msg: "Initialize a new clean apparmor.d build directory",
|
|
},
|
|
Path: "",
|
|
})
|
|
}
|
|
|
|
func (p Synchronise) Apply() ([]string, error) {
|
|
res := []string{}
|
|
dirs := paths.PathList{prebuild.RootApparmord, prebuild.Root.Join("root"), prebuild.Root.Join("systemd")}
|
|
for _, dir := range dirs {
|
|
if err := dir.RemoveAll(); err != nil {
|
|
return res, err
|
|
}
|
|
}
|
|
if p.Path == "" {
|
|
for _, name := range []string{"apparmor.d", "root"} {
|
|
if err := util.CopyTo(paths.New(name), prebuild.Root.Join(name)); err != nil {
|
|
return res, err
|
|
}
|
|
}
|
|
} else {
|
|
file := paths.New(p.Path)
|
|
destination, err := file.RelFrom(paths.New("apparmor.d"))
|
|
if err != nil {
|
|
return res, err
|
|
}
|
|
destination = prebuild.RootApparmord.JoinPath(destination)
|
|
if err := destination.Parent().MkdirAll(); err != nil {
|
|
return res, err
|
|
}
|
|
if err := file.CopyTo(destination); err != nil {
|
|
return res, err
|
|
}
|
|
res = append(res, destination.String())
|
|
}
|
|
return res, nil
|
|
}
|