mirror of
https://gitlab.com/apparmor/apparmor.git
synced 2025-03-08 18:31:03 +01:00
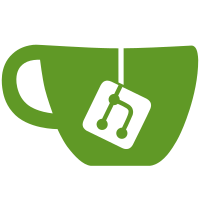
the openSUSE 10.2 RC candidates. This is because the _syscallN macros are (apparently) no longer user visible. This patch replaces uses of _syscallN() in the regression test source with invocations of syscall(2), the preferred linux kernel way of doing things. With this patch, our regression tests compile on the openSUSE 10.2 candidates as well as older distributions (tested as far back as slackware 10.0, which includes a 2.4.x kernel). (A missing license header got added as well as some minor coding style cleanups leaked into the patch as well.)
62 lines
1.2 KiB
C
62 lines
1.2 KiB
C
/* $Id:$ */
|
|
|
|
/*
|
|
* Copyright (C) 2006 Novell/SUSE
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation, version 2 of the
|
|
* License.
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include <sys/types.h>
|
|
#include <unistd.h>
|
|
#include <linux/unistd.h>
|
|
#include <stdlib.h>
|
|
#include <pthread.h>
|
|
#include <errno.h>
|
|
#include <sys/syscall.h>
|
|
#include "changehat.h"
|
|
|
|
void static inline dump_me(const char *msg)
|
|
{
|
|
//printf("(%d/%ld/0x%lx) %s\n", getpid(), syscall(SYS_gettid), pthread_self(), msg);
|
|
}
|
|
|
|
static int is_done;
|
|
static int thread_rc;
|
|
|
|
void *worker (void *param)
|
|
{
|
|
char *file = (char *) param;
|
|
int rc;
|
|
|
|
dump_me("worker called, changing hat");
|
|
change_hat ("fez", 12345);
|
|
rc = do_open(file);
|
|
dump_me("worker changed hat");
|
|
change_hat (NULL, 12345);
|
|
if (rc == 0) {
|
|
printf("PASS\n");
|
|
}
|
|
thread_rc = rc;
|
|
is_done = 1;
|
|
return 0;
|
|
}
|
|
|
|
int main (int argc, char **argv)
|
|
{
|
|
pthread_t child_process;
|
|
if (argc != 2) {
|
|
fprintf(stderr, "FAIL: usage '%s <filename>'\n", argv[0]);
|
|
exit(1);
|
|
}
|
|
|
|
dump_me("main");
|
|
pthread_create(&child_process, NULL, worker, argv[1]);
|
|
while (!is_done) {
|
|
sleep(1);
|
|
}
|
|
exit(thread_rc);
|
|
}
|