mirror of
https://gitlab.com/apparmor/apparmor.git
synced 2025-03-04 08:24:42 +01:00
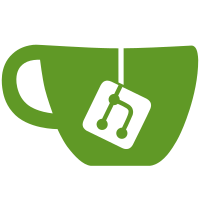
Differential state compression encodes a state's transitions as the difference between the state and its default state (the state it is relative too). This reduces the number of transitions that need to be stored in the transition table, hence reducing the size of the dfa. There is a trade off in that a single input character may have to traverse more than one state. This is somewhat offset by reduced table sizes providing better locality and caching properties. With carefully encoding we can still make constant match time guarentees. This patch guarentees that a state that is differentially encoded will do at most 3m state traversal to match an input of length m (as opposed to a non-differentially compressed dfa doing exactly m state traversals). In practice the actually number of extra traversals is less than this becaus we selectively choose which states are differentially encoded. In addition to reducing the size of the dfa by reducing the number of transitions that have to be stored. Differential encoding reduces the number of transitions that need to be considered by comb compression, which can result in tighter packing, due to a reduction in sparseness, and also reduces the time spent in comb compression which currently uses an O(n^2) algorithm. Differential encoding will always result in a DFA that is smaller or equal in size to the encoded DFA, and will usually improve compilation times, with the performance improvements increasing as the DFA gets larger. Eg. Given a example DFA that created 8991 states after minimization. * If only comb compression (current default) is used 52057 transitions are packed into a table of 69591 entries. Achieving an efficiency of about 75% (an average of about 7.74 table entries per state). With a resulting compressed dfa16 size of 404238 bytes and a run time for the dfa compilation of real 0m9.037s user 0m8.893s sys 0m0.036s * If differential encoding + comb compression is used, 8292 of the 8991 states are differentially encoded, with 31557 trans removed. Resulting in 20500 transitions are packed into a table of 20675 entries. Acheiving an efficiency of about 99.2% (an average of about 2.3 table entries per state With a resulting compressed dfa16 size of 207874 bytes (about 48.6% reduction) and a run time for the dfa compilation of real 0m5.416s (about 40% faster) user 0m5.280s sys 0m0.040s Repeating with a larger DFA that has 17033 states after minimization. * If only comb compression (current default) is used 102992 transitions are packed into a table of 137987 entries. Achieving an efficiency of about 75% (an average of about 8.10 entries per state). With a resultant compressed dfa16 size of 790410 bytes and a run time for d compilation of real 0m28.153s user 0m27.634s sys 0m0.120s * with differential encoding 39374 transition are packed into a table of 39594 entries. Achieving an efficiency of about 99.4% (an average of about 2.32 entries per state). With a resultant compressed dfa16 size of 396838 bytes (about 50% reduction and a run time for dfa compilation of real 0m11.804s (about 58% faster) user 0m11.657s sys 0m0.084s Signed-off-by: John Johansen <john.johansen@canonical.com> Acked-by: Seth Arnold <seth.arnold@canonical.com>
42 lines
792 B
C
42 lines
792 B
C
#ifndef __FLEX_TABLES_H
|
|
#define __FLEX_TABLES_H
|
|
|
|
#include <stdlib.h>
|
|
#include <stdint.h>
|
|
|
|
#define YYTH_MAGIC 0xF13C57B1
|
|
#define YYTH_FLAG_DIFF_ENCODE 1
|
|
|
|
struct table_set_header {
|
|
uint32_t th_magic; /* TH_MAGIC */
|
|
uint32_t th_hsize;
|
|
uint32_t th_ssize;
|
|
uint16_t th_flags;
|
|
/* char th_version[];
|
|
char th_name[];
|
|
char th_pad64[];*/
|
|
} __attribute__ ((packed));
|
|
|
|
#define YYTD_ID_ACCEPT 1
|
|
#define YYTD_ID_BASE 2
|
|
#define YYTD_ID_CHK 3
|
|
#define YYTD_ID_DEF 4
|
|
#define YYTD_ID_EC 5
|
|
#define YYTD_ID_META 6
|
|
#define YYTD_ID_ACCEPT2 7
|
|
#define YYTD_ID_NXT 8
|
|
|
|
#define YYTD_DATA8 1
|
|
#define YYTD_DATA16 2
|
|
#define YYTD_DATA32 4
|
|
|
|
struct table_header {
|
|
uint16_t td_id;
|
|
uint16_t td_flags;
|
|
uint32_t td_hilen;
|
|
uint32_t td_lolen;
|
|
/* char td_data[];
|
|
char td_pad64[];*/
|
|
} __attribute__ ((packed));
|
|
|
|
#endif
|