mirror of
https://gitlab.com/apparmor/apparmor.git
synced 2025-03-04 16:35:02 +01:00
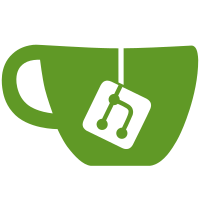
This patch implements the parsing of DBus rules. It attempts to catch all corner cases, such as specifying a bind permission with an interface conditional or specifying a subject name conditional and a peer name conditional in the same rule. It introduces the concept of conditional lists to the lexer and parser in order to handle 'peer=(label=/usr/bin/foo name=com.foo.bar)', since the existing list support in the lexer only supports a list of values. The DBus rules are encoded as follows: bus,name<bind_perm>,peer_label,path,interface,member<rw_perms> Bind rules stop matching at name<bind_perm>. Note that name is used for the subject name in bind rules and the peer name in rw rules. The function new_dbus_entry() is what does the proper sanitization to make sure that if a name conditional is specified, that it is the subject name in the case of a bind rule or that it is the peer name in the case of a rw rule. Signed-off-by: Tyler Hicks <tyhicks@canonical.com> Acked-by: Seth Arnold <seth.arnold@canonical.com>
48 lines
1.3 KiB
C
48 lines
1.3 KiB
C
/*
|
|
* Copyright (c) 2013
|
|
* Canonical, Ltd. (All rights reserved)
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of version 2 of the GNU General Public
|
|
* License published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, contact Novell, Inc. or Canonical
|
|
* Ltd.
|
|
*/
|
|
|
|
#ifndef __AA_DBUS_H
|
|
#define __AA_DBUS_H
|
|
|
|
#include "parser.h"
|
|
|
|
struct dbus_entry {
|
|
char *bus;
|
|
/**
|
|
* Be careful! ->name can be the subject or the peer name, depending on
|
|
* whether the rule is a bind rule or a send/receive rule. See the
|
|
* comments in new_dbus_entry() for details.
|
|
*/
|
|
char *name;
|
|
char *peer_label;
|
|
char *path;
|
|
char *interface;
|
|
char *member;
|
|
int mode;
|
|
int audit;
|
|
int deny;
|
|
|
|
struct dbus_entry *next;
|
|
};
|
|
|
|
void free_dbus_entry(struct dbus_entry *ent);
|
|
struct dbus_entry *new_dbus_entry(int mode, struct cond_entry *conds,
|
|
struct cond_entry *peer_conds);
|
|
void print_dbus_entry(struct dbus_entry *ent);
|
|
|
|
#endif /* __AA_DBUS_H */
|