mirror of
https://gitlab.com/apparmor/apparmor.git
synced 2025-03-04 08:24:42 +01:00
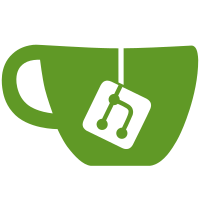
This enables adding a priority to a rules in policy, finishing out the priority work done to plumb priority support through the internals in the previous patch. Rules have a default priority of 0. The priority prefix can be added before the other currently support rule prefixes, ie. [priority prefix][audit qualifier][rule mode][owner] If present a numerical priority can be assigned to the rule, where the greater the number the higher the priority. Eg. priority=1 audit file r /etc/passwd, priority=-1 deny file w /etc/**, Rule priority allows the rule with the highest priority to completely override lower priority rules where they overlap. Within a given priority level rules will accumulate in standard apparmor fashion. Eg. given priority=1 w /*c, priority=0 r /a*, priority=-1 k /*b*, /abc, /bc, /ac .. will have permissions of w /ab, /abb, /aaa, .. will have permissions of r /b, /bcb, /bab, .. will have permissions of k User specified rule priorities are currently capped at the arbitrary values of 1000, and -1000. Notes: * not all rule types support the priority prefix. Rukes like - network - capability - rlimits need to be reworked need to be reworked to properly preserve the policy rule structure. * this patch does not support priority on rule blocks * this patch does not support using a variable in the priority value. Signed-off-by: John Johansen <john.johansen@canonical.com>
72 lines
1.9 KiB
C++
72 lines
1.9 KiB
C++
/*
|
|
* Copyright (c) 2023
|
|
* Canonical Ltd. (All rights reserved)
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of version 2 of the GNU General Public
|
|
* License published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, contact Canonical Ltd.
|
|
*/
|
|
|
|
#ifndef __AA_ALL_H
|
|
#define __AA_ALL_H
|
|
|
|
#include "rule.h"
|
|
|
|
#define AA_IO_URING_OVERRIDE_CREDS AA_MAY_APPEND
|
|
#define AA_IO_URING_SQPOLL AA_MAY_CREATE
|
|
|
|
#define AA_VALID_IO_URING_PERMS (AA_IO_URING_OVERRIDE_CREDS | \
|
|
AA_IO_URING_SQPOLL)
|
|
|
|
class all_rule: public prefix_rule_t {
|
|
void move_conditionals(struct cond_entry *conds);
|
|
public:
|
|
all_rule(void): prefix_rule_t(RULE_TYPE_ALL) { }
|
|
|
|
virtual bool valid_prefix(const prefixes &p, const char *&error) {
|
|
if (p.priority != 0) {
|
|
error = _("priority prefix not allowed on all rules");
|
|
return false;
|
|
}
|
|
if (p.owner) {
|
|
error = _("owner prefix not allowed on all rules");
|
|
return false;
|
|
}
|
|
return true;
|
|
};
|
|
|
|
int expand_variables(void)
|
|
{
|
|
return 0;
|
|
}
|
|
virtual ostream &dump(ostream &os) {
|
|
prefix_rule_t::dump(os);
|
|
|
|
os << "all";
|
|
|
|
return os;
|
|
}
|
|
virtual bool is_mergeable(void) { return true; }
|
|
virtual int cmp(rule_t const &rhs) const
|
|
{
|
|
return prefix_rule_t::cmp(rhs);
|
|
};
|
|
|
|
virtual void add_implied_rules(Profile &prof);
|
|
|
|
virtual int gen_policy_re(Profile &prof unused) { return RULE_OK; };
|
|
|
|
protected:
|
|
virtual void warn_once(const char *name unused, const char *msg unused) { };
|
|
virtual void warn_once(const char *name unused) { };
|
|
};
|
|
|
|
#endif /* __AA_ALL_H */
|