mirror of
https://gitlab.com/apparmor/apparmor.git
synced 2025-03-04 16:35:02 +01:00
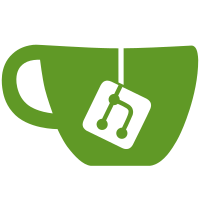
Introduce an apparmor.aa.init_aa() method and move the initialization code of the apparmor.aa module into it. Note that this change will break any external users of apparmor.aa because global variables that were previously initialized when importing apparmor.aa will not be initialized unless a call to the new apparmor.aa.init_aa() method is made. The main purpose of this change is to allow the utils tests to be able to set a non-default location for configuration files. Instead of hard-coding the location of logprof.conf and other utils related configuration files to /etc/apparmor/, this patch allows it to be configured by calling apparmor.aa.init_aa(confdir=PATH). This allows for the make check target to use the in-tree config file, profiles, and parser by default. A helper method, setup_aa(), is added to common_test.py that checks for an environment variable containing a non-default configuration directory path prior to calling apparmor.aa.init_aa(). All test scripts that use apparmor.aa are updated to call setup_aa(). Signed-off-by: Tyler Hicks <tyhicks@canonical.com> Suggested-by: Christian Boltz <apparmor@cboltz.de> Acked-by: Seth Arnold <seth.arnold@canonical.com> Acked-by: Christian Boltz <apparmor@cboltz.de>
47 lines
1.5 KiB
Python
47 lines
1.5 KiB
Python
#! /usr/bin/python3
|
|
# ------------------------------------------------------------------
|
|
#
|
|
# Copyright (C) 2014 Canonical Ltd.
|
|
#
|
|
# This program is free software; you can redistribute it and/or
|
|
# modify it under the terms of version 2 of the GNU General Public
|
|
# License published by the Free Software Foundation.
|
|
#
|
|
# ------------------------------------------------------------------
|
|
|
|
import apparmor.aa as aa
|
|
import unittest
|
|
from common_test import AAParseTest, setup_regex_tests, setup_aa
|
|
|
|
class BaseAAParseMountTest(AAParseTest):
|
|
def setUp(self):
|
|
self.parse_function = aa.parse_mount_rule
|
|
|
|
class AAParseMountTest(BaseAAParseMountTest):
|
|
tests = [
|
|
('mount,', 'mount base keyword rule'),
|
|
('mount -o ro,', 'mount ro rule'),
|
|
('mount -o rw /dev/sdb1 -> /mnt/external,', 'mount rw with mount point'),
|
|
]
|
|
|
|
class AAParseRemountTest(BaseAAParseMountTest):
|
|
tests = [
|
|
('remount,', 'remount base keyword rule'),
|
|
('remount -o ro,', 'remount ro rule'),
|
|
('remount -o ro /,', 'remount ro with mountpoint'),
|
|
]
|
|
|
|
class AAParseUmountTest(BaseAAParseMountTest):
|
|
tests = [
|
|
('umount,', 'umount base keyword rule'),
|
|
('umount /mnt/external,', 'umount with mount point'),
|
|
('unmount,', 'unmount base keyword rule'),
|
|
('unmount /mnt/external,', 'unmount with mount point'),
|
|
]
|
|
|
|
setup_aa(aa)
|
|
if __name__ == '__main__':
|
|
setup_regex_tests(AAParseMountTest)
|
|
setup_regex_tests(AAParseRemountTest)
|
|
setup_regex_tests(AAParseUmountTest)
|
|
unittest.main(verbosity=2)
|