mirror of
https://gitlab.com/apparmor/apparmor.git
synced 2025-03-04 16:35:02 +01:00
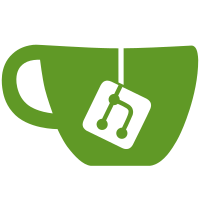
Tag start of entries in the policydb as being mediated. This makes the start state for any class being mediated be none 0. The kernel can detect this to determine whether the parser expected mediation for the class. This is just a way of encoding what features expect mediation within the policydb it self so that a separate table isn't needed. This is also used to indicate the new unix semantics for mediation of unix domain sockets on connect should be applied. Note: this does cause a fail open on situation on Ubuntu Saucy, which did not properly indicate support. That is if a kernel using this patch is installed on an Ubuntu Saucy system, unix domain socket mediation on connect won't happen, instead the older behavior will be applied. This won't cause policy failures as it is less strict than what Ubuntu Saucy applies. This is necessary so that AppArmor can properly function on older userspaces without a compile time configuration on the kernel to determine behavior. A kernel expecting this behavior will function correctly with all old userspaces expect it will not enforce connect time mediation on Ubuntu Saucy. However Ubuntu does not support Trusty (or newer) kernels as backports to Saucy, so this does not break them. Signed-off-by: John Johansen <john.johansen@canonical.com> Acked-by: Seth Arnold <seth.arnold@canonical.com>
76 lines
2.3 KiB
C
76 lines
2.3 KiB
C
/*
|
|
* Copyright (c) 2010 - 2012
|
|
* Canonical Ltd. (All rights reserved)
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of version 2 of the GNU General Public
|
|
* License published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, contact Novell, Inc. or Canonical,
|
|
* Ltd.
|
|
*/
|
|
#include <stdlib.h>
|
|
#include <stdarg.h>
|
|
#include <libintl.h>
|
|
#include <locale.h>
|
|
#define _(s) gettext(s)
|
|
#include "parser.h"
|
|
|
|
int perms_create = 0; /* perms contain create flag */
|
|
int net_af_max_override = -1; /* use kernel to determine af_max */
|
|
int kernel_load = 1;
|
|
int kernel_policy_version = 5; /* default to base version */
|
|
int kernel_supports_network = 0; /* kernel supports network rules */
|
|
int kernel_supports_policydb = 0; /* kernel supports new policydb */
|
|
int kernel_supports_mount = 0; /* kernel supports mount rules */
|
|
int kernel_supports_dbus = 0; /* kernel supports dbus rules */
|
|
int conf_verbose = 0;
|
|
int conf_quiet = 0;
|
|
int names_only = 0;
|
|
int current_lineno = 1;
|
|
int option = OPTION_ADD;
|
|
|
|
dfaflags_t dfaflags = (dfaflags_t)(DFA_CONTROL_TREE_NORMAL | DFA_CONTROL_TREE_SIMPLE | DFA_CONTROL_MINIMIZE );
|
|
|
|
char *subdomainbase = NULL;
|
|
const char *progname = __FILE__;
|
|
char *profile_ns = NULL;
|
|
char *profilename = NULL;
|
|
char *current_filename = NULL;
|
|
|
|
FILE *ofile = NULL;
|
|
|
|
#ifdef FORCE_READ_IMPLIES_EXEC
|
|
int read_implies_exec = 1;
|
|
#else
|
|
int read_implies_exec = 0;
|
|
#endif
|
|
|
|
void pwarn(const char *fmt, ...)
|
|
{
|
|
va_list arg;
|
|
char *newfmt;
|
|
|
|
if (conf_quiet || names_only || option == OPTION_REMOVE)
|
|
return;
|
|
|
|
if (asprintf(&newfmt, _("Warning from %s (%s%sline %d): %s"),
|
|
profilename ? profilename : "stdin",
|
|
current_filename ? current_filename : "",
|
|
current_filename ? " " : "",
|
|
current_lineno,
|
|
fmt) == -1)
|
|
return;
|
|
|
|
va_start(arg, fmt);
|
|
vfprintf(stderr, newfmt, arg);
|
|
va_end(arg);
|
|
|
|
free(newfmt);
|
|
}
|