mirror of
https://gitlab.com/apparmor/apparmor.git
synced 2025-03-04 08:24:42 +01:00
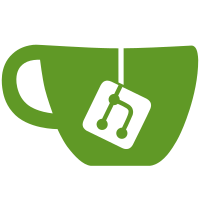
Construction of the chfa can reorder states from what the numbering given during the hfa constuctions because of reordering for better compression, dead state removal to ensure better packing etc. This however means the dfa dump is difficult (it is possible using multiple dumpes) to match up to the chfa that the kernel is using. Make this easier by making the dfa dump be able to take the emapping as input, and provide an option to dump the chfa equivalent hfa. Renumbered states will show up as {new <== {orig}} in the dump Eg. --D dfa-states {1} <== priority (allow/deny/prompt/audit/quiet) {5} 0 (0x 4/0//0/0/0) {1} perms: none 0x2 -> {5} 0 (0x 4/0//0/0/0) 0x4 -> {5} 0 (0x 4/0//0/0/0) \a 0x7 -> {5} 0 (0x 4/0//0/0/0) \t 0x9 -> {5} 0 (0x 4/0//0/0/0) \n 0xa -> {5} 0 (0x 4/0//0/0/0) \ 0x20 -> {5} 0 (0x 4/0//0/0/0) 4 0x34 -> {3} {3} perms: none 0x0 -> {6} {6} perms: none 1 0x31 -> {5} 0 (0x 4/0//0/0/0) -D dfa-compressed-states {1} <== priority (allow/deny/prompt/audit/quiet) {2 == {5}} 0 (0x 4/0//0/0/0) {1} perms: none 0x2 -> {2 == {5}} 0 (0x 4/0//0/0/0) 0x4 -> {2 == {5}} 0 (0x 4/0//0/0/0) \a 0x7 -> {2 == {5}} 0 (0x 4/0//0/0/0) \t 0x9 -> {2 == {5}} 0 (0x 4/0//0/0/0) \n 0xa -> {2 == {5}} 0 (0x 4/0//0/0/0) \ 0x20 -> {2 == {5}} 0 (0x 4/0//0/0/0) 4 0x34 -> {3} {3} perms: none 0x0 -> {4 == {6}} {4 == {6}} perms: none 1 0x31 -> {2 == {5}} 0 (0x 4/0//0/0/0) Signed-off-by: John Johansen <john.johansen@canonical.com>
74 lines
2.2 KiB
C++
74 lines
2.2 KiB
C++
/*
|
|
* (C) 2006, 2007 Andreas Gruenbacher <agruen@suse.de>
|
|
* Copyright (c) 2003-2008 Novell, Inc. (All rights reserved)
|
|
* Copyright 2009-2012 Canonical Ltd.
|
|
*
|
|
* The libapparmor library is licensed under the terms of the GNU
|
|
* Lesser General Public License, version 2.1. Please see the file
|
|
* COPYING.LGPL.
|
|
*
|
|
* This library is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU Lesser General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU Lesser General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*
|
|
*
|
|
* Create a compressed hfa (chfa) from an hfa
|
|
*/
|
|
#ifndef __LIBAA_RE_CHFA_H
|
|
#define __LIBAA_RE_CHFA_H
|
|
|
|
#include <map>
|
|
#include <vector>
|
|
|
|
#include "hfa.h"
|
|
#include "../perms.h"
|
|
|
|
#define BASE32_FLAGS 0xff000000
|
|
#define DiffEncodeBit32 0x80000000
|
|
#define MATCH_FLAG_OOB_TRANSITION 0x20000000
|
|
#define base_mask_size(X) ((X) & ~BASE32_FLAGS)
|
|
|
|
using namespace std;
|
|
|
|
typedef vector<pair<const State *, size_t> > DefaultBase;
|
|
typedef vector<pair<const State *, const State *> > NextCheck;
|
|
|
|
class CHFA {
|
|
public:
|
|
CHFA(void);
|
|
CHFA(DFA &dfa, map<transchar, transchar> &eq, optflags const &opts,
|
|
bool permindex, bool prompt);
|
|
void dump(ostream & os);
|
|
void flex_table(ostream &os, optflags const &opts);
|
|
void init_free_list(vector<pair<size_t, size_t> > &free_list,
|
|
size_t prev, size_t start);
|
|
bool fits_in(vector<pair<size_t, size_t> > &free_list, size_t base,
|
|
StateTrans &cases);
|
|
void insert_state(vector<pair<size_t, size_t> > &free_list,
|
|
State *state, DFA &dfa);
|
|
void weld_file_to_policy(CHFA &file_chfa, size_t &new_start,
|
|
bool accept_idx, bool prompt,
|
|
vector <aa_perms> &policy_perms,
|
|
vector <aa_perms> &file_perms);
|
|
|
|
// private:
|
|
// sigh templates suck, friend declaration does not work so for now
|
|
// make these public
|
|
vector<uint32_t> accept;
|
|
vector<uint32_t> accept2;
|
|
DefaultBase default_base;
|
|
NextCheck next_check;
|
|
const State *start;
|
|
Renumber_Map num;
|
|
map<transchar, transchar> eq;
|
|
unsigned int chfaflags;
|
|
private:
|
|
transchar max_eq;
|
|
ssize_t first_free;
|
|
};
|
|
|
|
#endif /* __LIBAA_RE_CHFA_H */
|