mirror of
https://gitlab.com/apparmor/apparmor.git
synced 2025-03-04 08:24:42 +01:00
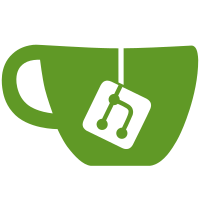
From: Goldwyn Rodrigues <rgoldwyn@suse.com> Provides json support to tools in order to interact with other utilities such as Yast. The JSON output is one per line, in order to differentiate between multiple records. Each JSON record has a "dialog" entry which defines the type of message passed. A response must contain the "dialog" entry. "info" message does not require a response. "apparmor-json-version" added in order to identify the communication protocol version for future updates. This is based on work done by Christian Boltz. Signed-off-by: Goldwyn Rodrigues <rgoldwyn@suse.com> Acked-by: Christian Boltz <apparmor@cboltz.de> Acked-by: Seth Arnold <seth.arnold@canonical.com>
57 lines
2 KiB
Python
Executable file
57 lines
2 KiB
Python
Executable file
#! /usr/bin/python3
|
|
# ----------------------------------------------------------------------
|
|
# Copyright (C) 2013 Kshitij Gupta <kgupta8592@gmail.com>
|
|
#
|
|
# This program is free software; you can redistribute it and/or
|
|
# modify it under the terms of version 2 of the GNU General Public
|
|
# License as published by the Free Software Foundation.
|
|
#
|
|
# This program is distributed in the hope that it will be useful,
|
|
# but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
# MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
# GNU General Public License for more details.
|
|
#
|
|
# ----------------------------------------------------------------------
|
|
import argparse
|
|
import os
|
|
|
|
import apparmor.aa as apparmor
|
|
import apparmor.ui as aaui
|
|
|
|
# setup exception handling
|
|
from apparmor.fail import enable_aa_exception_handler
|
|
enable_aa_exception_handler()
|
|
|
|
# setup module translations
|
|
from apparmor.translations import init_translation
|
|
_ = init_translation()
|
|
|
|
parser = argparse.ArgumentParser(description=_('Process log entries to generate profiles'))
|
|
parser.add_argument('-d', '--dir', type=str, help=_('path to profiles'))
|
|
parser.add_argument('-f', '--file', type=str, help=_('path to logfile'))
|
|
parser.add_argument('-m', '--mark', type=str, help=_('mark in the log to start processing after'))
|
|
parser.add_argument('-j', '--json', action='store_true', help=_('Input and Output in JSON'))
|
|
args = parser.parse_args()
|
|
|
|
if args.json:
|
|
aaui.set_json_mode()
|
|
|
|
profiledir = args.dir
|
|
logmark = args.mark or ''
|
|
|
|
apparmor.init_aa()
|
|
apparmor.set_logfile(args.file)
|
|
|
|
aa_mountpoint = apparmor.check_for_apparmor()
|
|
if not aa_mountpoint:
|
|
raise apparmor.AppArmorException(_('It seems AppArmor was not started. Please enable AppArmor and try again.'))
|
|
|
|
if profiledir:
|
|
apparmor.profile_dir = apparmor.get_full_path(profiledir)
|
|
if not os.path.isdir(apparmor.profile_dir):
|
|
raise apparmor.AppArmorException("%s is not a directory."%profiledir)
|
|
|
|
apparmor.loadincludes()
|
|
|
|
apparmor.do_logprof_pass(logmark)
|
|
|