mirror of
https://github.com/evilsocket/opensnitch.git
synced 2025-03-06 09:30:58 +01:00
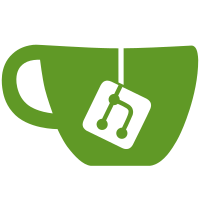
The server address and log file were hardcoded into the opensnitchd.service file, making it almost impossible to change. Soon we'll be able to change it from the UI.
108 lines
2.7 KiB
Go
108 lines
2.7 KiB
Go
package ui
|
|
|
|
import (
|
|
"encoding/json"
|
|
"fmt"
|
|
"io/ioutil"
|
|
"strings"
|
|
|
|
"github.com/gustavo-iniguez-goya/opensnitch/daemon/log"
|
|
"github.com/gustavo-iniguez-goya/opensnitch/daemon/procmon"
|
|
"github.com/gustavo-iniguez-goya/opensnitch/daemon/rule"
|
|
)
|
|
|
|
func (c *Client) getSocketPath(socketPath string) string {
|
|
if strings.HasPrefix(socketPath, "unix://") == true {
|
|
c.isUnixSocket = true
|
|
return socketPath[7:]
|
|
}
|
|
|
|
c.isUnixSocket = false
|
|
return socketPath
|
|
}
|
|
|
|
func (c *Client) isProcMonitorEqual(newMonitorMethod string) bool {
|
|
config.RLock()
|
|
defer config.RUnlock()
|
|
|
|
return newMonitorMethod == config.ProcMonitorMethod
|
|
}
|
|
|
|
func (c *Client) parseConf(rawConfig string) (conf Config, err error) {
|
|
err = json.Unmarshal([]byte(rawConfig), &conf)
|
|
return conf, err
|
|
}
|
|
|
|
func (c *Client) loadDiskConfiguration(reload bool) {
|
|
raw, err := ioutil.ReadFile(configFile)
|
|
if err != nil {
|
|
fmt.Errorf("Error loading disk configuration %s: %s", configFile, err)
|
|
}
|
|
|
|
if ok := c.loadConfiguration(raw); ok {
|
|
if err := c.configWatcher.Add(configFile); err != nil {
|
|
log.Error("Could not watch path: %s", err)
|
|
return
|
|
}
|
|
}
|
|
|
|
if reload {
|
|
return
|
|
}
|
|
|
|
go c.monitorConfigWorker()
|
|
}
|
|
|
|
func (c *Client) loadConfiguration(rawConfig []byte) bool {
|
|
config.Lock()
|
|
defer config.Unlock()
|
|
|
|
if err := json.Unmarshal(rawConfig, &config); err != nil {
|
|
fmt.Errorf("Error parsing configuration %s: %s", configFile, err)
|
|
return false
|
|
}
|
|
// firstly load config level, to detect further errors if any
|
|
if config.LogLevel != nil {
|
|
log.SetLogLevel(int(*config.LogLevel))
|
|
}
|
|
|
|
if config.Server.Address != "" {
|
|
tempSocketPath := c.getSocketPath(config.Server.Address)
|
|
if tempSocketPath != c.socketPath {
|
|
// disconnect, and let the connection poller reconnect to the new address
|
|
c.disconnect()
|
|
}
|
|
c.socketPath = tempSocketPath
|
|
}
|
|
if config.Server.LogFile != "" {
|
|
if err := log.OpenFile(config.Server.LogFile); err != nil {
|
|
log.Warning("Error opening log file: ", err)
|
|
}
|
|
}
|
|
|
|
if config.DefaultAction != "" {
|
|
clientDisconnectedRule.Action = rule.Action(config.DefaultAction)
|
|
clientErrorRule.Action = rule.Action(config.DefaultAction)
|
|
}
|
|
if config.DefaultDuration != "" {
|
|
clientDisconnectedRule.Duration = rule.Duration(config.DefaultDuration)
|
|
clientErrorRule.Duration = rule.Duration(config.DefaultDuration)
|
|
}
|
|
if config.ProcMonitorMethod != "" {
|
|
procmon.SetMonitorMethod(config.ProcMonitorMethod)
|
|
}
|
|
|
|
return true
|
|
}
|
|
|
|
func (c *Client) saveConfiguration(rawConfig string) (err error) {
|
|
if c.loadConfiguration([]byte(rawConfig)) != true {
|
|
return fmt.Errorf("Error parsing configuration %s: %s", rawConfig, err)
|
|
}
|
|
|
|
if err = ioutil.WriteFile(configFile, []byte(rawConfig), 0644); err != nil {
|
|
log.Error("writing configuration to disk: ", err)
|
|
return err
|
|
}
|
|
return nil
|
|
}
|