mirror of
https://github.com/evilsocket/opensnitch.git
synced 2025-03-04 16:44:46 +01:00
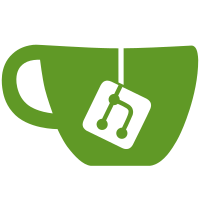
daemon tasks are actions that are executed in background by the daemon. They're started from the GUI (server) via a Notification (protobuf), with the type TASK_START (protobuf). Once received in the daemon, the TaskManager starts the task in background. Tasks may run at interval times (every 5s, 2days, etc), until they finish an operation, until a timeout, etc. Each task has each own configuration options, which will customize the behaviour of its operations. In this version, if the GUI is closed, the daemon will stop all the running tasks. Each Task has a flag to ignore this behaviour, for example if they need to run until they finish and only send a notification to the GUI, instead of streaming data continuously to the GUI (server). - Up until now we only had one task that could be initiated from the GUI: the process monitor dialog. It has been migrated to a Task{}. - go.mod bumped to v1.20, to use unsafe string functions. - go.sum updated accordingly.
55 lines
1.6 KiB
Go
55 lines
1.6 KiB
Go
package tasks
|
|
|
|
import (
|
|
"context"
|
|
)
|
|
|
|
// TaskBase holds the common fields of every task.
|
|
// Warning: don't define fields in tasks with these names.
|
|
type TaskBase struct {
|
|
Ctx context.Context
|
|
Cancel context.CancelFunc
|
|
Results chan interface{}
|
|
Errors chan error
|
|
StopChan chan struct{}
|
|
|
|
// Stop the task if the daemon is disconnected from the GUI (server).
|
|
// Some tasks don't need to run if the daemon is not connected to the GUI,
|
|
// like PIDMonitor, SocketsMonitor,etc.
|
|
// There might be other tasks that will perform some actions, and they
|
|
// may send a notification on finish.
|
|
StopOnDisconnect bool
|
|
}
|
|
|
|
// Task defines the interface for tasks that the task manager will execute.
|
|
type Task interface {
|
|
// Start starts the task, potentially running it asynchronously.
|
|
Start(ctx context.Context, cancel context.CancelFunc) error
|
|
|
|
// Stop stops the task.
|
|
Stop() error
|
|
|
|
Pause() error
|
|
Resume() error
|
|
|
|
// Results returns a channel that can be used to receive task results.
|
|
Results() <-chan interface{}
|
|
|
|
// channel used to send errors
|
|
Errors() <-chan error
|
|
}
|
|
|
|
// TaskNotification is the data we receive when a new task is started from
|
|
// the GUI (server).
|
|
// The notification.data field will contain a string like:
|
|
// '{"name": "...", "data": {"interval": "3s", "...": ...} }'
|
|
//
|
|
// where Name is the task to start, sa defined by the Name var of each task,
|
|
// and Data is the configuration of each task (a map[string]string, converted by the json package).
|
|
type TaskNotification struct {
|
|
// Data of the task.
|
|
Data map[string]string
|
|
|
|
// Name of the task.
|
|
Name string
|
|
}
|