mirror of
https://github.com/evilsocket/opensnitch.git
synced 2025-03-04 08:34:40 +01:00
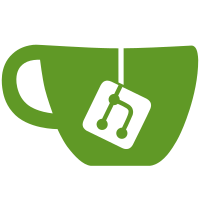
Use the timestamp instead of the event object when iterating over the last events. ~15x speed increase. Increase event buffer to 100. On my machine I routinely hit the ceiling of 50 events under some multitasking workloads. Small buffer results in connection attempts not being logged. Fix Makefile to rebuild when ui.proto changes
32 lines
681 B
Go
32 lines
681 B
Go
package statistics
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/evilsocket/opensnitch/daemon/conman"
|
|
"github.com/evilsocket/opensnitch/daemon/rule"
|
|
"github.com/evilsocket/opensnitch/daemon/ui/protocol"
|
|
)
|
|
|
|
type Event struct {
|
|
Time time.Time
|
|
Connection *conman.Connection
|
|
Rule *rule.Rule
|
|
}
|
|
|
|
func NewEvent(con *conman.Connection, match *rule.Rule) *Event {
|
|
return &Event{
|
|
Time: time.Now(),
|
|
Connection: con,
|
|
Rule: match,
|
|
}
|
|
}
|
|
|
|
func (e *Event) Serialize() *protocol.Event {
|
|
return &protocol.Event{
|
|
Time: e.Time.Format("2006-01-02 15:04:05"),
|
|
Connection: e.Connection.Serialize(),
|
|
Rule: e.Rule.Serialize(),
|
|
Unixnano: e.Time.UnixNano(),
|
|
}
|
|
}
|