mirror of
https://github.com/evilsocket/opensnitch.git
synced 2025-03-06 01:20:59 +01:00
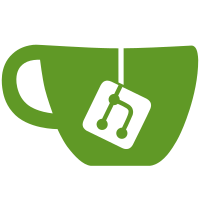
problem: - after losing network connectivity node<->server, the node didn't restore the connection. In reality, the connection with the server was not closed, but the notifications channel was closed due to inactivity after 20s. set inactivity timeouts to 20s on both node and server. Previous timeouts were 2h for the main connection and 20s for the streaming channels (notifications). - get rid of the logic to determine if the server is alive or not based on sending pings. Instead, use the connection events when a node connects/disconnects (Subscribe). The Ping call is still used to send the statistics. other: - fixed exception when updating the status of a node.
92 lines
3.3 KiB
Python
Executable file
92 lines
3.3 KiB
Python
Executable file
#!/usr/bin/env python3
|
|
|
|
from PyQt5 import QtWidgets, QtGui, QtCore
|
|
|
|
import sys
|
|
import os
|
|
import time
|
|
import signal
|
|
import argparse
|
|
import logging
|
|
|
|
logging.getLogger().disabled = True
|
|
|
|
from concurrent import futures
|
|
|
|
import grpc
|
|
|
|
dist_path = '/usr/lib/python3/dist-packages/'
|
|
if dist_path not in sys.path:
|
|
sys.path.append(dist_path)
|
|
|
|
from opensnitch.service import UIService
|
|
from opensnitch.config import Config
|
|
import opensnitch.version
|
|
import opensnitch.ui_pb2
|
|
from opensnitch.ui_pb2_grpc import add_UIServicer_to_server
|
|
|
|
def on_exit():
|
|
app.quit()
|
|
server.stop(0)
|
|
sys.exit(0)
|
|
|
|
def supported_qt_version(major, medium, minor):
|
|
q = QtCore.QT_VERSION_STR.split(".")
|
|
return int(q[0]) >= major and int(q[1]) >= medium and int(q[2]) >= minor
|
|
|
|
if __name__ == '__main__':
|
|
parser = argparse.ArgumentParser(description='OpenSnitch UI service.')
|
|
parser.add_argument("--socket", dest="socket", default="unix:///tmp/osui.sock", help="Path of the unix socket for the gRPC service (https://github.com/grpc/grpc/blob/master/doc/naming.md).", metavar="FILE")
|
|
parser.add_argument("--max-clients", dest="serverWorkers", default=10, help="Max number of allowed clients (incoming connections).")
|
|
|
|
args = parser.parse_args()
|
|
|
|
os.environ["QT_AUTO_SCREEN_SCALE_FACTOR"] = "1"
|
|
if supported_qt_version(5,6,0):
|
|
try:
|
|
# NOTE: maybe we also need Qt::AA_UseHighDpiPixmaps
|
|
QtCore.QApplication.setAttribute(QtCore.Qt.AA_EnableHighDpiScaling, True)
|
|
except Exception:
|
|
pass
|
|
|
|
locale = QtCore.QLocale.system()
|
|
i18n_path = os.path.dirname(os.path.realpath(opensnitch.__file__)) + "/i18n"
|
|
print("Loading translations:", i18n_path, "locale:", locale.name())
|
|
translator = QtCore.QTranslator()
|
|
translator.load(i18n_path + "/" + locale.name() + "/opensnitch-" + locale.name() + ".qm")
|
|
app = QtWidgets.QApplication(sys.argv)
|
|
app.installTranslator(translator)
|
|
|
|
service = UIService(app, on_exit)
|
|
# @doc: https://grpc.github.io/grpc/python/grpc.html#server-object
|
|
server = grpc.server(futures.ThreadPoolExecutor(),
|
|
options=(
|
|
# https://github.com/grpc/grpc/blob/master/doc/keepalive.md
|
|
# https://grpc.github.io/grpc/core/group__grpc__arg__keys.html
|
|
# send keepalive ping every 5 second, default is 2 hours)
|
|
('grpc.keepalive_time_ms', 5000),
|
|
# after 5s of inactivity, wait 20s and close the connection if
|
|
# there's no response.
|
|
('grpc.keepalive_timeout_ms', 20000),
|
|
('grpc.keepalive_permit_without_calls', True),
|
|
))
|
|
|
|
add_UIServicer_to_server(service, server)
|
|
|
|
if args.socket.startswith("unix://"):
|
|
socket = args.socket[7:]
|
|
socket = os.path.abspath(socket)
|
|
server.add_insecure_port("unix:%s" % socket)
|
|
else:
|
|
server.add_insecure_port(args.socket)
|
|
|
|
# https://stackoverflow.com/questions/5160577/ctrl-c-doesnt-work-with-pyqt
|
|
signal.signal(signal.SIGINT, signal.SIG_DFL)
|
|
|
|
try:
|
|
# print "OpenSnitch UI service running on %s ..." % socket
|
|
server.start()
|
|
app.exec_()
|
|
except KeyboardInterrupt:
|
|
on_exit()
|
|
|