mirror of
https://github.com/evilsocket/opensnitch.git
synced 2025-03-06 09:30:58 +01:00
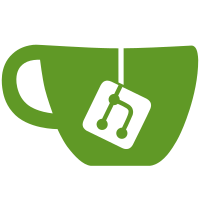
Use auditd events to keep a list of PIDs which open sockets, reading them from the audisp af_unix plugin. - Install auditd and audisp-plugins - Enable the af_unix plugin (/etc/audisp-plugin/af_unix, active = yes) - Start opensnitch with -process-monitor-method audit. If the choosen method is audit but it's not active or not installed, it'll fallback to /proc anyway. If it's properly configured, a debug trace will be written to the logs: "PID found via audit events ..."
45 lines
858 B
Go
45 lines
858 B
Go
package procmon
|
|
|
|
import (
|
|
"github.com/gustavo-iniguez-goya/opensnitch/daemon/log"
|
|
"github.com/gustavo-iniguez-goya/opensnitch/daemon/procmon/audit"
|
|
)
|
|
|
|
type Process struct {
|
|
ID int
|
|
Path string
|
|
Args []string
|
|
Env map[string]string
|
|
}
|
|
|
|
func NewProcess(pid int, path string) *Process {
|
|
return &Process{
|
|
ID: pid,
|
|
Path: path,
|
|
Args: make([]string, 0),
|
|
Env: make(map[string]string),
|
|
}
|
|
}
|
|
|
|
func End() {
|
|
if MonitorMethod == MethodAudit {
|
|
audit.Stop()
|
|
} else if MonitorMethod == MethodFtrace {
|
|
go Stop()
|
|
}
|
|
}
|
|
|
|
func Init() {
|
|
if MonitorMethod == MethodFtrace {
|
|
if err := Start(); err == nil {
|
|
return
|
|
}
|
|
} else if MonitorMethod == MethodAudit {
|
|
if c, err := audit.Start(); err == nil {
|
|
go audit.Reader(c, (chan<- audit.Event)(audit.EventChan))
|
|
return
|
|
}
|
|
}
|
|
log.Info("Process monitor parsing /proc")
|
|
MonitorMethod = MethodProc
|
|
}
|