mirror of
https://github.com/capnproto/pycapnp.git
synced 2025-03-04 16:35:04 +01:00
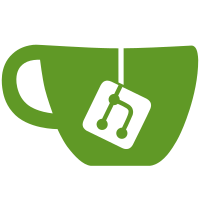
Note: I've tried not to break any behaviour of the previously working APIs Python API Changes / Additions - capnp/lib/capnp.pyx * class _RemotePromise + [Added] cpdef _wait(self) = Exception raising code that used to be inside of wait(self) + [Modified] def wait(self) = Same functionality as before + [Added] async def a_wait(self) = Cannot use await as that's a reserved keyword = Uses pollRemote and asyncio.sleep(0) to make call asynchronous * class _TwoPartyVatNetwork + [Added] cdef _init_pipe(self, _TwoWayPipe pipe, Side side, schema_cpp.ReaderOptions opts) = Instanciates a TwoPartyVatNetwork using a TwoWayPipe (instead of using a file handle or connection as before) * class TwoPartyClient + [Modified] def __init__(self, socket=None, restorer=None, traversal_limit_in_words=None, nesting_limit=None) = Changes the socket parameter to be optional = If socket is not specified, default to using a TwoWayPipe + [Added] async def read(self, bufsize) = awaitable function that blocks until data has been read = bufsize defines the maximum amount of data to be read back (e.g. 4096 bytes) = Reads data from TwoWayPipe + [Added] def write(self, data) = Write data to TwoWayPipe = Not awaitable as the write interface of the TwoWayPipe doesn't have poll functionality * class TwoPartyServer + [Modified] def __init__(self, socket=None, restorer=None, server_socket=None, bootstrap=None, traversal_limit_in_words=None, nesting_limit=None) = Changes the socket parameter to be optional = If socket is not specified, default to using a TwoWayPipe = Simplified code by removing an else (self._connect) + [Added] async def read(self, bufsize) = awaitable function that blocks until data has been read = bufsize defines the maximum amount of data to be read back (e.g. 4096 bytes) = Reads data from TwoWayPipe + [Added] def write(self, data) = Write data to TwoWayPipe = Not awaitable as the write interface of the TwoWayPipe doesn't have poll functionality + [Added] async def poll_forever(self) = asyncio equivalent of run_forever() * class _TwoWayPipe + Wrapper class for TwoWayPipe Other Additions - capnp/helpers/asyncHelper.h * pollWaitScope + Pumps the kj event handler + Used for the TwoWayServer * pollRemote + Polls a remote promise + i.e. a capnp RPC call - capnp/helpers/asyncIoHelper.h * AsyncIoStreamReadHelper + I wasn't able to figure out Promise[size_t] using Cython so this was the next best thing I could think of doing + Was needed to handle read polling from a read promise = Polling is used for asyncio as kj waits need a wrapper to be compatible - capnp/lib/capnp.pyx * makeTwoWayPipe + Wrapper for kj newTwoWayPipe function * poll_once + Single pump of the kj event handler (used with pollWaitScope) TwoWayClient Usage - TwoWayPipe - See examples/async_client.py TwoWayServer Usage - TwoWayPipe - See examples/async_server.py capnp/helpers/asyncIoHelper.h Misc Changes - Fixed thread_server.py and thread_client.py to use bootstrap instead of ez_restore - async_client.py and async_server.py examples * Uses the same thread.capnp as thread_client.py and thread_server.py * They are compatible, so you can mix and match client and server for compatibility testing * async_client.py and async_server.py require <address>:<port> formatting (unlike autodetection from thread_client.py and thread_server.py)
53 lines
999 B
C++
53 lines
999 B
C++
#pragma once
|
|
|
|
#include "kj/async.h"
|
|
#include "kj/async-io.h"
|
|
|
|
class AsyncIoStreamReadHelper {
|
|
public:
|
|
AsyncIoStreamReadHelper(kj::AsyncIoStream * _stream, kj::WaitScope * _scope, size_t bufsize) {
|
|
io_stream = _stream;
|
|
wait_scope = _scope;
|
|
ready = false;
|
|
buffer_read_size = 0;
|
|
buffer = new unsigned char[bufsize];
|
|
promise = io_stream->read(buffer, 1, bufsize);
|
|
}
|
|
|
|
~AsyncIoStreamReadHelper() {
|
|
delete[] buffer;
|
|
}
|
|
|
|
bool poll() {
|
|
bool result = promise.poll(*wait_scope);
|
|
if (result) {
|
|
ready = true;
|
|
buffer_read_size = promise.wait(*wait_scope);
|
|
}
|
|
return result;
|
|
}
|
|
|
|
size_t read_size() {
|
|
if (!ready) {
|
|
return 0;
|
|
}
|
|
return buffer_read_size;
|
|
}
|
|
|
|
void * read_buffer() {
|
|
if (!ready) {
|
|
return 0;
|
|
}
|
|
return buffer;
|
|
}
|
|
|
|
private:
|
|
kj::AsyncIoStream * io_stream;
|
|
kj::WaitScope * wait_scope;
|
|
kj::Promise<size_t> promise = nullptr;
|
|
|
|
unsigned char *buffer;
|
|
size_t buffer_read_size;
|
|
|
|
bool ready;
|
|
};
|