mirror of
https://github.com/capnproto/pycapnp.git
synced 2025-03-04 16:35:04 +01:00
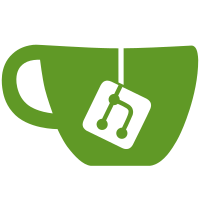
* Integrate the KJ event loop into Python's asyncio event loop Fix #256 This PR attempts to remove the slow and expensive polling behavior for asyncio in favor of proper linking of the KJ event loop to the asyncio event loop. * Don't memcopy buffer * Improve promise cancellation and prepare for timer implementation * Add attribution for asyncProvider.cpp * Implement timeout * Cleanup * First round of simplifications * Add more a_wait functions and a shutdown function * Fix edge-cases with loop shutdown * Clean up calculator examples * Cleanup * Cleanup * Reformat * Fix warnings * Reformat again * Compatibility with macos * Inline the asyncio loop in some places where this is feasible * Add todo * Fix * Remove synchronous wait * Wrap fd listening callbacks in a class * Remove poll_forever * Remove the thread-local/thread-global optimization This will not matter much soon anyway, and simplifies things * Share promise code by using fused types * Improve refcounting of python objects in promises We replace many instances of PyObject* by Own<PyRefCounter> for more automatic reference management. * Code wrapPyFunc in a similar way to wrapPyFuncNoArg * Refactor capabilityHelper, fix several memory bugs for promises and add __await__ * Improve promise ownership, reduce memory leaks Promise wrappers now hold a Own<Promise<Own<PyRefCounter>>> object. This might seem like excessive nesting of objects (which to some degree it is, but with good reason): - The outer Own is needed because Cython cannot allocate objects without a nullary constructor on the stack (Promise doesn't have a nullary constructor). Additionally, I believe it would be difficult or impossible to detect when a promise is cancelled/moved if we use a bare Promise. - Every promise returns a Owned PyRefCounter. PyRefCounter makes sure that a reference to the returned object keeps existing until the promise is fulfilled or cancelled. Previously, this was attempted using attach, which is redundant and makes reasoning about PyINCREF and PyDECREF very difficult. - Because a promise holds a Own<Promise<...>>, when we perform any kind of action on that promise (a_wait, then, ...), we have to explicitly move() the ownership around. This will leave the original promise with a NULL-pointer, which we can easily detect as a cancelled promise. Promises now only hold references to their 'parents' when strictly needed. This should reduce memory pressure. * Simplify and test the promise joining functionality * Attach forgotten parent * Catch exceptions in add_reader and friends * Further cleanup of memory leaks * Get rid of a_wait() in examples * Cancel all fd read operations when the python asyncio loop is closed * Formatting * Remove support for capnp < 7000 * Bring asyncProvider.cpp more in line with upstream async-io-unix.c++ It was originally copied from the nodejs implementation, which in turn copied from async-io-unix.c++. But that copy is pretty old. * Fix a bug that caused file descriptors to never be closed * Implement AsyncIoStream based on Python transports and protocols * Get rid of asyncProvider All asyncio now goes through _AsyncIoStream * Formatting * Add __dict__ to PyAsyncIoStreamProtocol for python 3.7 * Reintroduce strange ipv4/ipv6 selection code to make ci happy * Extra pause_reading() * Work around more python bugs * Be careful to only close transport when this is still possible * Move pause_reading() workaround
13 lines
419 B
C
13 lines
419 B
C
#pragma once
|
|
|
|
#include "kj/async.h"
|
|
#include "capabilityHelper.h"
|
|
|
|
void waitNeverDone(kj::WaitScope & scope) {
|
|
kj::NEVER_DONE.wait(scope);
|
|
}
|
|
|
|
capnp::Response< ::capnp::DynamicStruct> * waitRemote(kj::Own<capnp::RemotePromise<::capnp::DynamicStruct>> promise,
|
|
kj::WaitScope & scope) {
|
|
return new capnp::Response< ::capnp::DynamicStruct>(promise->wait(scope));
|
|
}
|