mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 08:24:40 +01:00
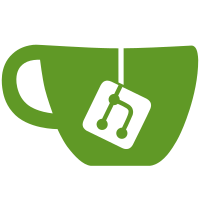
* refactor: project requires 3.9+ changed as per NEP-29 https://numpy.org/neps/nep-0029-deprecation_policy.html * test: nose type tests are deprecated in pytest now * fix: deprecation of ast.Str and ast.Bytes and .s attribute access * fix: deprecation of ast.Num,ast.NameConstant,ast.Ellipsis * refactor: upgrade code to be py39+ using ruff the changes are auto-generated * refactor: remove typing.Annotated compatibility code * fix: temporarily disable having xonsh syntax inside f-strings * test: skip failing tests there is no workaround for this version. It might get solved in the final release though * refactor: make XonshSession.completer lazily populated this speedsup the tests as cmd_cache would not be actively populated when used in default_completers function * refactor: make presence of walrus operator default
88 lines
3.2 KiB
Python
88 lines
3.2 KiB
Python
import os
|
|
import tempfile
|
|
from unittest import TestCase
|
|
|
|
from xonsh.tools import ON_WINDOWS
|
|
from xonsh.xoreutils import _which
|
|
|
|
|
|
class TestWhich(TestCase):
|
|
# Tests for the _whichgen function which is the only thing we
|
|
# use from the _which.py module.
|
|
def setUp(self):
|
|
# Setup two folders with some test files.
|
|
self.testdirs = [tempfile.TemporaryDirectory(), tempfile.TemporaryDirectory()]
|
|
if ON_WINDOWS:
|
|
self.testapps = ["whichtestapp1.exe", "whichtestapp2.wta"]
|
|
self.exts = [".EXE"]
|
|
else:
|
|
self.testapps = ["whichtestapp1"]
|
|
self.exts = None
|
|
for app in self.testapps:
|
|
for d in self.testdirs:
|
|
path = os.path.join(d.name, app)
|
|
with open(path, "wb") as f:
|
|
f.write(b"")
|
|
os.chmod(path, 0o755)
|
|
|
|
def tearDown(self):
|
|
for d in self.testdirs:
|
|
d.cleanup()
|
|
|
|
def test_whichgen(self):
|
|
testdir = self.testdirs[0].name
|
|
arg = "whichtestapp1"
|
|
matches = list(_which.whichgen(arg, path=[testdir], exts=self.exts))
|
|
assert len(matches) == 1
|
|
assert self._file_match(matches[0][0], os.path.join(testdir, arg))
|
|
|
|
def test_whichgen_failure(self):
|
|
testdir = self.testdirs[0].name
|
|
arg = "not_a_file"
|
|
matches = list(_which.whichgen(arg, path=[testdir], exts=self.exts))
|
|
assert len(matches) == 0
|
|
|
|
def test_whichgen_verbose(self):
|
|
testdir = self.testdirs[0].name
|
|
arg = "whichtestapp1"
|
|
matches = list(
|
|
_which.whichgen(arg, path=[testdir], exts=self.exts, verbose=True)
|
|
)
|
|
assert len(matches) == 1
|
|
match, from_where = matches[0]
|
|
assert self._file_match(match, os.path.join(testdir, arg))
|
|
assert from_where == "from given path element 0"
|
|
|
|
def test_whichgen_multiple(self):
|
|
testdir0 = self.testdirs[0].name
|
|
testdir1 = self.testdirs[1].name
|
|
arg = "whichtestapp1"
|
|
matches = list(_which.whichgen(arg, path=[testdir0, testdir1], exts=self.exts))
|
|
assert len(matches) == 2
|
|
assert self._file_match(matches[0][0], os.path.join(testdir0, arg))
|
|
assert self._file_match(matches[1][0], os.path.join(testdir1, arg))
|
|
|
|
if ON_WINDOWS:
|
|
|
|
def test_whichgen_ext_failure(self):
|
|
testdir = self.testdirs[0].name
|
|
arg = "whichtestapp2"
|
|
matches = list(_which.whichgen(arg, path=[testdir], exts=self.exts))
|
|
assert len(matches) == 0
|
|
|
|
def test_whichgen_ext_success(self):
|
|
testdir = self.testdirs[0].name
|
|
arg = "whichtestapp2"
|
|
matches = list(_which.whichgen(arg, path=[testdir], exts=[".wta"]))
|
|
assert len(matches) == 1
|
|
assert self._file_match(matches[0][0], os.path.join(testdir, arg))
|
|
|
|
def _file_match(self, path1, path2):
|
|
if ON_WINDOWS:
|
|
path1 = os.path.normpath(os.path.normcase(path1))
|
|
path2 = os.path.normpath(os.path.normcase(path2))
|
|
path1 = os.path.splitext(path1)[0]
|
|
path2 = os.path.splitext(path2)[0]
|
|
return path1 == path2
|
|
else:
|
|
return os.path.samefile(path1, path2)
|