mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 16:34:47 +01:00
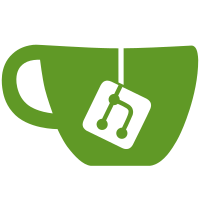
I wouldn't normally do something like this but issue #487 brought to my attention the fact that too many of the python modules don't have an encoding comment and of those that do there is a lot of pointless inconsistency in the style of the comment.
66 lines
1.7 KiB
Python
66 lines
1.7 KiB
Python
# -*- coding: utf-8 -*-
|
|
"""Tests the xonsh lexer."""
|
|
from __future__ import unicode_literals, print_function
|
|
import sys
|
|
import glob
|
|
import builtins
|
|
import platform
|
|
import subprocess
|
|
from contextlib import contextmanager
|
|
|
|
from nose.plugins.skip import SkipTest
|
|
|
|
from xonsh.built_ins import ensure_list_of_strs
|
|
|
|
|
|
VER_3_4 = (3, 4)
|
|
VER_3_5 = (3, 5)
|
|
VER_MAJOR_MINOR = sys.version_info[:2]
|
|
ON_MAC = (platform.system() == 'Darwin')
|
|
|
|
def sp(cmd):
|
|
return subprocess.check_output(cmd, universal_newlines=True)
|
|
|
|
@contextmanager
|
|
def mock_xonsh_env(xenv):
|
|
builtins.__xonsh_env__ = xenv
|
|
builtins.__xonsh_help__ = lambda x: x
|
|
builtins.__xonsh_glob__ = glob.glob
|
|
builtins.__xonsh_exit__ = False
|
|
builtins.__xonsh_superhelp__ = lambda x: x
|
|
builtins.__xonsh_regexpath__ = lambda x: []
|
|
builtins.__xonsh_subproc_captured__ = sp
|
|
builtins.__xonsh_subproc_uncaptured__ = sp
|
|
builtins.__xonsh_ensure_list_of_strs__ = ensure_list_of_strs
|
|
builtins.evalx = None
|
|
builtins.execx = None
|
|
builtins.compilex = None
|
|
builtins.aliases = {}
|
|
yield
|
|
del builtins.__xonsh_env__
|
|
del builtins.__xonsh_help__
|
|
del builtins.__xonsh_glob__
|
|
del builtins.__xonsh_exit__
|
|
del builtins.__xonsh_superhelp__
|
|
del builtins.__xonsh_regexpath__
|
|
del builtins.__xonsh_subproc_captured__
|
|
del builtins.__xonsh_subproc_uncaptured__
|
|
del builtins.__xonsh_ensure_list_of_strs__
|
|
del builtins.evalx
|
|
del builtins.execx
|
|
del builtins.compilex
|
|
del builtins.aliases
|
|
|
|
|
|
def skipper():
|
|
"""Raises SkipTest"""
|
|
raise SkipTest
|
|
|
|
def skip_if(cond):
|
|
"""Skips a test under a given condition."""
|
|
def dec(f):
|
|
if cond:
|
|
return skipper
|
|
else:
|
|
return f
|
|
return dec
|