mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 16:34:47 +01:00
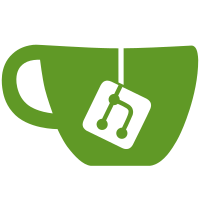
* add test for importing empty .xsh file * test: empty lines do not get appended to history prompt-toolkit needs its own test outside of test_base_shell.py because it uses a custom _push() method * fix: do not append empty/comment-only input to history Adds a compile_empty_tree argument to Execer.compile() By default, the argument is `True`, and `compile()` returns a compiled `pass` statement for comment-only input. When the argument is `False`, `compile()` returns `None` for comment-only input. The base shell and prompt-toolkit shell use `compile_empty_tree = False` so that they get `None` as the compiled code and don't append the command to the history. * add news * fix tests
70 lines
1.4 KiB
Python
70 lines
1.4 KiB
Python
"""Testing xonsh import hooks"""
|
|
import os
|
|
from importlib import import_module
|
|
|
|
import pytest
|
|
|
|
from xonsh import imphooks
|
|
|
|
|
|
@pytest.fixture(autouse=True)
|
|
def imp_env(xession):
|
|
xession.env.update({"PATH": [], "PATHEXT": []})
|
|
imphooks.install_import_hooks(xession.execer)
|
|
yield
|
|
|
|
|
|
def test_import():
|
|
import sample
|
|
|
|
assert "hello mom jawaka\n" == sample.x
|
|
|
|
|
|
def test_import_empty():
|
|
from xpack import empty_xsh
|
|
|
|
assert empty_xsh
|
|
|
|
|
|
def test_absolute_import():
|
|
from xpack import sample
|
|
|
|
assert "hello mom jawaka\n" == sample.x
|
|
|
|
|
|
def test_relative_import():
|
|
from xpack import relimp
|
|
|
|
assert "hello mom jawaka\n" == relimp.sample.x
|
|
assert "hello mom jawaka\ndark chest of wonders" == relimp.y
|
|
|
|
|
|
def test_sub_import():
|
|
from xpack.sub import sample
|
|
|
|
assert "hello mom jawaka\n" == sample.x
|
|
|
|
|
|
TEST_DIR = os.path.dirname(__file__)
|
|
|
|
|
|
def test_module_dunder_file_attribute():
|
|
import sample
|
|
|
|
exp = os.path.join(TEST_DIR, "sample.xsh")
|
|
assert os.path.abspath(sample.__file__) == exp
|
|
|
|
|
|
def test_module_dunder_file_attribute_sub():
|
|
from xpack.sub import sample
|
|
|
|
exp = os.path.join(TEST_DIR, "xpack", "sub", "sample.xsh")
|
|
assert os.path.abspath(sample.__file__) == exp
|
|
|
|
|
|
def test_get_source():
|
|
mod = import_module("sample")
|
|
loader = mod.__loader__
|
|
source = loader.get_source("sample")
|
|
with open(os.path.join(TEST_DIR, "sample.xsh")) as srcfile:
|
|
assert source == srcfile.read()
|