mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 08:24:40 +01:00
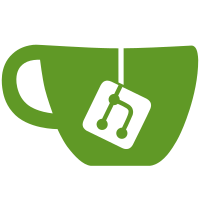
* feat: use github actions to deploy docs fixes #4473 * fix: install xonsh * fix: doc action * chore: support *.md for documentation * fix: remove hardcoded docs link it affects local testing * refactor: move sphinx extensions to its own package * fix: remove deprecated get_theme_dir As of Sphinx 1.2, this is passed to Sphinx via a ``setup.py`` entry point, and no longer needs to be included in your documentation's ``conf.py``. * feat: jinja2 render without affecting incremental build * style: * feat: auto generate API doc no need to create a file for each module * chore: watch top-level and nested files * refactor: update lib api-docs as well * fix: import errors when doc generated for some modules * fix: relative path * feat: add release handling * Update .github/workflows/docs.yml Co-authored-by: Gil Forsyth <gforsyth@users.noreply.github.com> * refactor: use github-app-token * chore: deploy docs only when merged Co-authored-by: Gil Forsyth <gforsyth@users.noreply.github.com>
67 lines
2 KiB
Python
67 lines
2 KiB
Python
"""This module adds a reST directive to sphinx that generates alias
|
|
documentation. For example::
|
|
|
|
.. command-help:: xonsh.aliases.source_foreign
|
|
|
|
.. command-help:: xonsh.aliases.source_foreign -h
|
|
|
|
will create help for aliases.
|
|
"""
|
|
import importlib
|
|
import io
|
|
import textwrap
|
|
|
|
from docutils import nodes
|
|
|
|
try:
|
|
from docutils.utils.error_reporting import ErrorString # the new way
|
|
except ImportError:
|
|
from docutils.error_reporting import ErrorString # the old way
|
|
from docutils.parsers.rst import Directive
|
|
from docutils.statemachine import ViewList
|
|
|
|
from sphinx.util.nodes import nested_parse_with_titles
|
|
|
|
from xonsh.tools import redirect_stdout, redirect_stderr
|
|
|
|
|
|
class CommandHelp(Directive):
|
|
"""The command-help directive, which is based on constructing a list of
|
|
of string lines of restructured text and then parsing it into its own node.
|
|
Note that this will add the '--help' flag automatically.
|
|
"""
|
|
|
|
required_arguments = 1
|
|
optional_arguments = 1
|
|
final_argument_whitespace = True
|
|
option_spec = {}
|
|
has_content = False
|
|
|
|
def run(self):
|
|
arguments = self.arguments
|
|
lines = [".. code-block:: none", ""]
|
|
m, f = arguments[0].rsplit(".", 1)
|
|
mod = importlib.import_module(m)
|
|
func = getattr(mod, f)
|
|
args = ["--help"] if len(arguments) == 1 else arguments[1:]
|
|
stdout = io.StringIO()
|
|
stderr = io.StringIO()
|
|
with redirect_stdout(stdout), redirect_stderr(stderr):
|
|
try:
|
|
func(args)
|
|
except SystemExit:
|
|
pass
|
|
stdout.seek(0)
|
|
s = stdout.read()
|
|
lines += textwrap.indent(s, " ").splitlines()
|
|
|
|
# hook to docutils
|
|
src, lineno = self.state_machine.get_source_and_line(self.lineno)
|
|
vl = ViewList(lines, source=src)
|
|
node = nodes.paragraph()
|
|
nested_parse_with_titles(self.state, vl, node)
|
|
return node.children
|
|
|
|
|
|
def setup(app):
|
|
app.add_directive("command-help", CommandHelp)
|