mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 08:24:40 +01:00
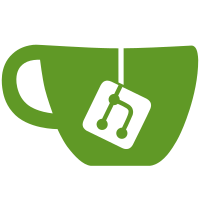
* refactor: remove usage of global variables in abbrevs.py * chore: add flake8-mutable to prevent mutable defaults * fix: abbrevs expand test * refactor: add xonsh session singleton * refactor: fix circular errors when using xonshSession as singleton * refactor: remove black magicked builtin attributes * style: black format tests as well * refactor: update tests to use xonsh-session singleton * refactor: update abbrevs to not use builtins * test: remove DummyCommandsCache and patch orig class * fix: failing test_command_completers * test: use monkeypatch to update xession fixture * fix: failing test_pipelines * fix: failing test_main * chore: run test suit as single invocation * test: fix tests/test_xonsh.xsh * refactor: remove builtins from docs/conf.py * fix: mypy error in jobs * fix: test error from test_main * test: close xession error in test_command_completers * chore: use pytest-cov for reporting coverage this will include subprocess calls, and will increase coverage * style:
43 lines
1.5 KiB
Python
43 lines
1.5 KiB
Python
"""Jumping across whole words (non-whitespace) with Ctrl+Left/Right.
|
|
|
|
Alt+Left/Right remains unmodified to jump over smaller word segments.
|
|
"""
|
|
from prompt_toolkit.keys import Keys
|
|
|
|
from xonsh.built_ins import XSH
|
|
|
|
__all__ = ()
|
|
|
|
|
|
@XSH.builtins.events.on_ptk_create
|
|
def custom_keybindings(bindings, **kw):
|
|
|
|
# Key bindings for jumping over whole words (everything that's not
|
|
# white space) using Ctrl+Left and Ctrl+Right;
|
|
# Alt+Left and Alt+Right still jump over smaller word segments.
|
|
# See https://github.com/xonsh/xonsh/issues/2403
|
|
|
|
@bindings.add(Keys.ControlLeft)
|
|
def ctrl_left(event):
|
|
buff = event.current_buffer
|
|
pos = buff.document.find_previous_word_beginning(count=event.arg, WORD=True)
|
|
if pos:
|
|
buff.cursor_position += pos
|
|
|
|
@bindings.add(Keys.ControlRight)
|
|
def ctrl_right(event):
|
|
buff = event.current_buffer
|
|
pos = buff.document.find_next_word_ending(count=event.arg, WORD=True)
|
|
if pos:
|
|
buff.cursor_position += pos
|
|
|
|
@bindings.add(Keys.ShiftDelete)
|
|
def shift_delete(event):
|
|
buff = event.current_buffer
|
|
startpos, endpos = buff.document.find_boundaries_of_current_word(WORD=True)
|
|
startpos = buff.cursor_position + startpos - 1
|
|
startpos = 0 if startpos < 0 else startpos
|
|
endpos = buff.cursor_position + endpos
|
|
endpos = endpos + 1 if startpos == 0 else endpos
|
|
buff.text = buff.text[:startpos] + buff.text[endpos:]
|
|
buff.cursor_position = startpos
|