mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 08:24:40 +01:00
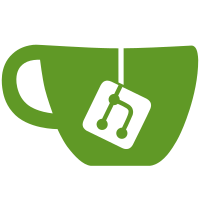
* add test for importing empty .xsh file * test: empty lines do not get appended to history prompt-toolkit needs its own test outside of test_base_shell.py because it uses a custom _push() method * fix: do not append empty/comment-only input to history Adds a compile_empty_tree argument to Execer.compile() By default, the argument is `True`, and `compile()` returns a compiled `pass` statement for comment-only input. When the argument is `False`, `compile()` returns `None` for comment-only input. The base shell and prompt-toolkit shell use `compile_empty_tree = False` so that they get `None` as the compiled code and don't append the command to the history. * add news * fix tests
65 lines
1.9 KiB
Python
65 lines
1.9 KiB
Python
"""(A down payment on) Testing for ``xonsh.base_shell.BaseShell`` and associated classes"""
|
|
import os
|
|
|
|
import pytest
|
|
|
|
from xonsh.base_shell import BaseShell
|
|
from xonsh.shell import transform_command
|
|
|
|
|
|
def test_pwd_tracks_cwd(xession, xonsh_execer, tmpdir_factory, monkeypatch):
|
|
asubdir = str(tmpdir_factory.mktemp("asubdir"))
|
|
cur_wd = os.getcwd()
|
|
xession.env.update(
|
|
dict(PWD=cur_wd, XONSH_CACHE_SCRIPTS=False, XONSH_CACHE_EVERYTHING=False)
|
|
)
|
|
|
|
monkeypatch.setattr(xonsh_execer, "cacheall", False, raising=False)
|
|
bc = BaseShell(xonsh_execer, None)
|
|
|
|
assert os.getcwd() == cur_wd
|
|
|
|
bc.default('os.chdir(r"' + asubdir + '")')
|
|
|
|
assert os.path.abspath(os.getcwd()) == os.path.abspath(asubdir)
|
|
assert os.path.abspath(os.getcwd()) == os.path.abspath(xession.env["PWD"])
|
|
assert "OLDPWD" in xession.env
|
|
assert os.path.abspath(cur_wd) == os.path.abspath(xession.env["OLDPWD"])
|
|
|
|
|
|
def test_transform(xession):
|
|
@xession.builtins.events.on_transform_command
|
|
def spam2egg(cmd, **_):
|
|
if cmd == "spam":
|
|
return "egg"
|
|
else:
|
|
return cmd
|
|
|
|
assert transform_command("spam") == "egg"
|
|
assert transform_command("egg") == "egg"
|
|
assert transform_command("foo") == "foo"
|
|
|
|
|
|
@pytest.mark.parametrize(
|
|
"cmd,exp_append_history",
|
|
[
|
|
("", False),
|
|
("# a comment", False),
|
|
("print('yes')", True),
|
|
],
|
|
)
|
|
def test_default_append_history(cmd, exp_append_history, xonsh_session, monkeypatch):
|
|
"""Test that running an empty line or a comment does not append to history"""
|
|
append_history_calls = []
|
|
|
|
def mock_append_history(**info):
|
|
append_history_calls.append(info)
|
|
|
|
monkeypatch.setattr(
|
|
xonsh_session.shell.shell, "_append_history", mock_append_history
|
|
)
|
|
xonsh_session.shell.default(cmd)
|
|
if exp_append_history:
|
|
assert len(append_history_calls) == 1
|
|
else:
|
|
assert len(append_history_calls) == 0
|