mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 16:34:47 +01:00
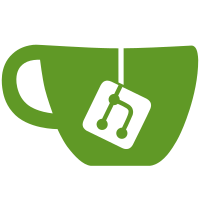
* refactor: remove usage of global variables in abbrevs.py * chore: add flake8-mutable to prevent mutable defaults * fix: abbrevs expand test * refactor: add xonsh session singleton * refactor: fix circular errors when using xonshSession as singleton * refactor: remove black magicked builtin attributes * style: black format tests as well * refactor: update tests to use xonsh-session singleton * refactor: update abbrevs to not use builtins * test: remove DummyCommandsCache and patch orig class * fix: failing test_command_completers * test: use monkeypatch to update xession fixture * fix: failing test_pipelines * fix: failing test_main * chore: run test suit as single invocation * test: fix tests/test_xonsh.xsh * refactor: remove builtins from docs/conf.py * fix: mypy error in jobs * fix: test error from test_main * test: close xession error in test_command_completers * chore: use pytest-cov for reporting coverage this will include subprocess calls, and will increase coverage * style:
158 lines
3.7 KiB
Python
158 lines
3.7 KiB
Python
import pytest
|
|
|
|
from tests.tools import skip_if_pre_3_8
|
|
from xonsh.completers.python import (
|
|
python_signature_complete,
|
|
complete_import,
|
|
complete_python,
|
|
)
|
|
from xonsh.parsers.completion_context import (
|
|
CommandArg,
|
|
CommandContext,
|
|
CompletionContext,
|
|
CompletionContextParser,
|
|
PythonContext,
|
|
)
|
|
|
|
|
|
@pytest.fixture(autouse=True)
|
|
def xonsh_execer_autouse(xession, xonsh_execer, monkeypatch):
|
|
monkeypatch.setitem(xession.env, "COMPLETIONS_BRACKETS", True)
|
|
return xonsh_execer
|
|
|
|
|
|
def foo(x, y, z):
|
|
pass
|
|
|
|
|
|
def bar(wakka="wow", jawaka="mom"):
|
|
pass
|
|
|
|
|
|
def baz(sonata, artica=True):
|
|
pass
|
|
|
|
|
|
def always_true(x, y):
|
|
return True
|
|
|
|
|
|
BASE_CTX = {"foo": foo, "bar": bar, "baz": baz}
|
|
FOO_ARGS = {"x=", "y=", "z="}
|
|
BAR_ARGS = {"wakka=", "jawaka="}
|
|
BAZ_ARGS = {"sonata=", "artica="}
|
|
|
|
|
|
@pytest.mark.parametrize(
|
|
"line, end, exp",
|
|
[
|
|
("foo(", 4, FOO_ARGS), # I have no idea why this one needs to be first
|
|
("foo()", 3, set()),
|
|
("foo()", 4, FOO_ARGS),
|
|
("foo()", 5, set()),
|
|
("foo(x, ", 6, FOO_ARGS),
|
|
("foo(x, )", 6, FOO_ARGS),
|
|
("bar()", 4, BAR_ARGS),
|
|
("baz()", 4, BAZ_ARGS),
|
|
("foo(bar(", 8, BAR_ARGS),
|
|
("foo(bar()", 9, FOO_ARGS),
|
|
("foo(bar())", 4, FOO_ARGS),
|
|
],
|
|
)
|
|
def test_complete_python_signatures(line, end, exp):
|
|
ctx = dict(BASE_CTX)
|
|
obs = python_signature_complete("", line, end, ctx, always_true)
|
|
assert exp == obs
|
|
|
|
|
|
@pytest.mark.parametrize(
|
|
"code, exp",
|
|
(
|
|
("x = su", "sum"),
|
|
("imp", "import"),
|
|
("{}.g", "{}.get("),
|
|
# no signature for native builtins under 3.7:
|
|
pytest.param("''.split(ma", "maxsplit=", marks=skip_if_pre_3_8),
|
|
),
|
|
)
|
|
def test_complete_python(code, exp):
|
|
res = complete_python(
|
|
CompletionContext(python=PythonContext(code, len(code), ctx={}))
|
|
)
|
|
assert res and len(res) == 2
|
|
comps, _ = res
|
|
assert exp in comps
|
|
|
|
|
|
def test_complete_python_ctx():
|
|
class A:
|
|
def wow(self):
|
|
pass
|
|
|
|
a = A()
|
|
|
|
res = complete_python(
|
|
CompletionContext(python=PythonContext("a.w", 2, ctx=locals()))
|
|
)
|
|
assert res and len(res) == 2
|
|
comps, _ = res
|
|
assert "a.wow(" in comps
|
|
|
|
|
|
@pytest.mark.parametrize(
|
|
"command, exp",
|
|
(
|
|
(
|
|
CommandContext(args=(CommandArg("import"),), arg_index=1, prefix="pathli"),
|
|
{"pathlib"},
|
|
),
|
|
(
|
|
CommandContext(args=(CommandArg("from"),), arg_index=1, prefix="pathli"),
|
|
{"pathlib "},
|
|
),
|
|
(
|
|
CommandContext(args=(CommandArg("import"),), arg_index=1, prefix="os.pa"),
|
|
{"os.path"},
|
|
),
|
|
(
|
|
CommandContext(
|
|
args=(
|
|
CommandArg("from"),
|
|
CommandArg("x"),
|
|
),
|
|
arg_index=2,
|
|
),
|
|
{"import"},
|
|
),
|
|
(
|
|
CommandContext(
|
|
args=(
|
|
CommandArg("import"),
|
|
CommandArg("os,"),
|
|
),
|
|
arg_index=2,
|
|
prefix="pathli",
|
|
),
|
|
{"pathlib"},
|
|
),
|
|
(
|
|
CommandContext(
|
|
args=(
|
|
CommandArg("from"),
|
|
CommandArg("pathlib"),
|
|
CommandArg("import"),
|
|
),
|
|
arg_index=3,
|
|
prefix="PurePa",
|
|
),
|
|
{"PurePath"},
|
|
),
|
|
),
|
|
)
|
|
def test_complete_import(command, exp):
|
|
result = complete_import(
|
|
CompletionContext(
|
|
command, python=PythonContext("", 0) # `complete_import` needs this
|
|
)
|
|
)
|
|
assert result == exp
|