mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 08:24:40 +01:00
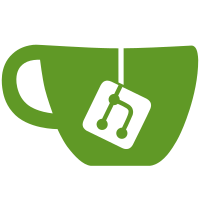
* delete package ptk; rename ptk2 to ptk_shell.; leave ptk2 as alias for ptk_shell. * SHELL_TYPE "prompt_toolkit" only; remove ptk1 specific behavior. * Doc updates: eliminate reference to prompt-toolkit < 2.0 * update requirements files ptk>=2; test shell_style="none" * fix ptk2 stub per code review * Add ptk2 to list of packages to install.
61 lines
1.9 KiB
Python
61 lines
1.9 KiB
Python
"""
|
|
Command abbreviations.
|
|
|
|
This expands input words from `abbrevs` disctionary as you type.
|
|
"""
|
|
|
|
import builtins
|
|
from prompt_toolkit.filters import completion_is_selected, IsMultiline
|
|
from prompt_toolkit.keys import Keys
|
|
from xonsh.built_ins import DynamicAccessProxy
|
|
from xonsh.tools import check_for_partial_string
|
|
|
|
__all__ = ()
|
|
|
|
builtins.__xonsh__.abbrevs = dict()
|
|
proxy = DynamicAccessProxy("abbrevs", "__xonsh__.abbrevs")
|
|
setattr(builtins, "abbrevs", proxy)
|
|
|
|
|
|
def expand_abbrev(buffer):
|
|
abbrevs = getattr(builtins, "abbrevs", None)
|
|
if abbrevs is None:
|
|
return
|
|
document = buffer.document
|
|
word = document.get_word_before_cursor(WORD=True)
|
|
if word in abbrevs.keys():
|
|
partial = document.text[: document.cursor_position]
|
|
startix, endix, quote = check_for_partial_string(partial)
|
|
if startix is not None and endix is None:
|
|
return
|
|
buffer.delete_before_cursor(count=len(word))
|
|
buffer.insert_text(abbrevs[word])
|
|
|
|
|
|
@events.on_ptk_create
|
|
def custom_keybindings(bindings, **kw):
|
|
|
|
from xonsh.ptk_shell.key_bindings import carriage_return
|
|
from prompt_toolkit.filters import EmacsInsertMode, ViInsertMode
|
|
|
|
handler = bindings.add
|
|
insert_mode = ViInsertMode() | EmacsInsertMode()
|
|
|
|
@handler(" ", filter=IsMultiline() & insert_mode)
|
|
def handle_space(event):
|
|
buffer = event.app.current_buffer
|
|
expand_abbrev(buffer)
|
|
buffer.insert_text(" ")
|
|
|
|
@handler(
|
|
Keys.ControlJ, filter=IsMultiline() & insert_mode & ~completion_is_selected
|
|
)
|
|
@handler(
|
|
Keys.ControlM, filter=IsMultiline() & insert_mode & ~completion_is_selected
|
|
)
|
|
def multiline_carriage_return(event):
|
|
buffer = event.app.current_buffer
|
|
current_char = buffer.document.current_char
|
|
if not current_char or current_char.isspace():
|
|
expand_abbrev(buffer)
|
|
carriage_return(buffer, event.cli)
|