mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 16:34:47 +01:00
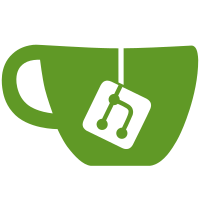
* feat: use github actions to deploy docs fixes #4473 * fix: install xonsh * fix: doc action * chore: support *.md for documentation * fix: remove hardcoded docs link it affects local testing * refactor: move sphinx extensions to its own package * fix: remove deprecated get_theme_dir As of Sphinx 1.2, this is passed to Sphinx via a ``setup.py`` entry point, and no longer needs to be included in your documentation's ``conf.py``. * feat: jinja2 render without affecting incremental build * style: * feat: auto generate API doc no need to create a file for each module * chore: watch top-level and nested files * refactor: update lib api-docs as well * fix: import errors when doc generated for some modules * fix: relative path * feat: add release handling * Update .github/workflows/docs.yml Co-authored-by: Gil Forsyth <gforsyth@users.noreply.github.com> * refactor: use github-app-token * chore: deploy docs only when merged Co-authored-by: Gil Forsyth <gforsyth@users.noreply.github.com>
43 lines
1,007 B
Python
43 lines
1,007 B
Python
"""ReST-Jinja2 helpers"""
|
|
import re
|
|
|
|
|
|
def underline_title(title: str, level: int = -1):
|
|
symbols = "-.^"
|
|
if level < len(symbols):
|
|
under = symbols[level]
|
|
else:
|
|
under = symbols[-1]
|
|
return under * len(title)
|
|
|
|
|
|
def to_valid_id(name: str) -> str:
|
|
return re.sub(r"[^\w]", "_", name.lower()).strip("_")
|
|
|
|
|
|
def to_valid_name(name: str) -> str:
|
|
return name.replace("`", "")
|
|
|
|
|
|
def indent_depth(depth: int = None):
|
|
return " " * ((1 if depth else 0) * 4)
|
|
|
|
|
|
def to_ref_string(title: str, underline=False, depth=-1) -> str:
|
|
title = str(title)
|
|
ref = f":ref:`{to_valid_name(title)} <{to_valid_id(title)}>`"
|
|
if underline:
|
|
return "\n".join([ref, underline_title(ref, depth)])
|
|
return ref
|
|
|
|
|
|
def iterator_for_divmod(iterable, div: int = 3):
|
|
items = list(iterable)
|
|
size = len(items)
|
|
rem = size % div
|
|
complement = size + div - rem
|
|
for i in range(complement):
|
|
if i < size:
|
|
yield items[i]
|
|
else:
|
|
yield None
|