mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 08:24:40 +01:00
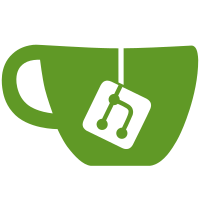
* refactor: remove usage of global variables in abbrevs.py * chore: add flake8-mutable to prevent mutable defaults * fix: abbrevs expand test * refactor: add xonsh session singleton * refactor: fix circular errors when using xonshSession as singleton * refactor: remove black magicked builtin attributes * style: black format tests as well * refactor: update tests to use xonsh-session singleton * refactor: update abbrevs to not use builtins * test: remove DummyCommandsCache and patch orig class * fix: failing test_command_completers * test: use monkeypatch to update xession fixture * fix: failing test_pipelines * fix: failing test_main * chore: run test suit as single invocation * test: fix tests/test_xonsh.xsh * refactor: remove builtins from docs/conf.py * fix: mypy error in jobs * fix: test error from test_main * test: close xession error in test_command_completers * chore: use pytest-cov for reporting coverage this will include subprocess calls, and will increase coverage * style:
40 lines
1.3 KiB
Python
40 lines
1.3 KiB
Python
# -*- coding: utf-8 -*-
|
|
"""(A down payment on) Testing for ``xonsh.base_shell.BaseShell`` and associated classes"""
|
|
import os
|
|
|
|
from xonsh.environ import Env
|
|
from xonsh.base_shell import BaseShell
|
|
from xonsh.shell import transform_command
|
|
|
|
|
|
def test_pwd_tracks_cwd(xession, xonsh_execer, tmpdir_factory, monkeypatch):
|
|
asubdir = str(tmpdir_factory.mktemp("asubdir"))
|
|
cur_wd = os.getcwd()
|
|
xession.env = Env(
|
|
PWD=cur_wd, XONSH_CACHE_SCRIPTS=False, XONSH_CACHE_EVERYTHING=False
|
|
)
|
|
|
|
monkeypatch.setattr(xonsh_execer, "cacheall", False, raising=False)
|
|
bc = BaseShell(xonsh_execer, None)
|
|
|
|
assert os.getcwd() == cur_wd
|
|
|
|
bc.default('os.chdir(r"' + asubdir + '")')
|
|
|
|
assert os.path.abspath(os.getcwd()) == os.path.abspath(asubdir)
|
|
assert os.path.abspath(os.getcwd()) == os.path.abspath(xession.env["PWD"])
|
|
assert "OLDPWD" in xession.env
|
|
assert os.path.abspath(cur_wd) == os.path.abspath(xession.env["OLDPWD"])
|
|
|
|
|
|
def test_transform(xession):
|
|
@xession.builtins.events.on_transform_command
|
|
def spam2egg(cmd, **_):
|
|
if cmd == "spam":
|
|
return "egg"
|
|
else:
|
|
return cmd
|
|
|
|
assert transform_command("spam") == "egg"
|
|
assert transform_command("egg") == "egg"
|
|
assert transform_command("foo") == "foo"
|