mirror of
https://github.com/xonsh/xonsh.git
synced 2025-03-04 08:24:40 +01:00
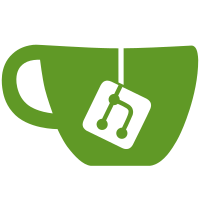
* feat: add function to make event registration from function signature * docs: add xontrib special functions description * feat: handle xontribs with special functions also reduce usage of XSH singleton * fix: missing XSH for now import singleton * docs: fix .rst format * fix: failing tests * feat: implement primitive xontrib-unload and xontrib-reload * chore: give explicit name * docs: update doc
45 lines
1.6 KiB
Python
45 lines
1.6 KiB
Python
"""Jumping across whole words (non-whitespace) with Ctrl+Left/Right.
|
|
|
|
Alt+Left/Right remains unmodified to jump over smaller word segments.
|
|
Shift+Delete removes the whole word.
|
|
"""
|
|
from prompt_toolkit.keys import Keys
|
|
|
|
from xonsh.built_ins import XonshSession
|
|
|
|
|
|
def custom_keybindings(bindings, **kw):
|
|
|
|
# Key bindings for jumping over whole words (everything that's not
|
|
# white space) using Ctrl+Left and Ctrl+Right;
|
|
# Alt+Left and Alt+Right still jump over smaller word segments.
|
|
# See https://github.com/xonsh/xonsh/issues/2403
|
|
|
|
@bindings.add(Keys.ControlLeft)
|
|
def ctrl_left(event):
|
|
buff = event.current_buffer
|
|
pos = buff.document.find_previous_word_beginning(count=event.arg, WORD=True)
|
|
if pos:
|
|
buff.cursor_position += pos
|
|
|
|
@bindings.add(Keys.ControlRight)
|
|
def ctrl_right(event):
|
|
buff = event.current_buffer
|
|
pos = buff.document.find_next_word_ending(count=event.arg, WORD=True)
|
|
if pos:
|
|
buff.cursor_position += pos
|
|
|
|
@bindings.add(Keys.ShiftDelete)
|
|
def shift_delete(event):
|
|
buff = event.current_buffer
|
|
startpos, endpos = buff.document.find_boundaries_of_current_word(WORD=True)
|
|
startpos = buff.cursor_position + startpos - 1
|
|
startpos = 0 if startpos < 0 else startpos
|
|
endpos = buff.cursor_position + endpos
|
|
endpos = endpos + 1 if startpos == 0 else endpos
|
|
buff.text = buff.text[:startpos] + buff.text[endpos:]
|
|
buff.cursor_position = startpos
|
|
|
|
|
|
def _load_xontrib_(xsh: XonshSession, **_):
|
|
xsh.builtins.events.on_ptk_create(custom_keybindings)
|