mirror of
https://git.pwmt.org/pwmt/zathura.git
synced 2024-09-21 15:41:18 +02:00
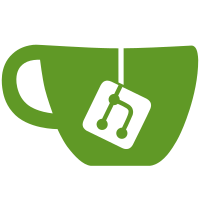
The plan is to put in adjustment.c every piece of code that has to do with document positioning, either computing it from data on the document side, or talking to GTK. We want to have at our disposal functions to compute sizes and positions without having to ask for it to a GTK widget. The new functions are: - move page_calc_height_width to adjustment.c - add page_calc_position that rotates a position relative to a page according to the rotation settings. - add position_to_page_number that computes the number of a page sitting at a given position (given in document-relative coordinates) - add page_number_to_position that computes the position (in document relative coordinates) that will be placed at the center of the viewport. - add page_is_visible that checks whether the given page intersects the viewport.
115 lines
3.8 KiB
C
115 lines
3.8 KiB
C
/* See LICENSE file for license and copyright information */
|
|
|
|
#ifndef ZATHURA_ADJUSTMENT_H
|
|
#define ZATHURA_ADJUSTMENT_H
|
|
|
|
#include <gtk/gtk.h>
|
|
#include <stdbool.h>
|
|
#include "document.h"
|
|
|
|
/**
|
|
* Calculate the page size according to the corrent scaling and rotation if
|
|
* desired.
|
|
*
|
|
* @param document the document
|
|
* @param height width the original height and width
|
|
* @return page_height page_width the scaled and rotated height and width
|
|
* @param rotate honor page's rotation
|
|
* @return real scale after rounding
|
|
*/
|
|
double page_calc_height_width(zathura_document_t* document, double height, double width,
|
|
unsigned int* page_height, unsigned int* page_width, bool rotate);
|
|
|
|
/**
|
|
* Calculate a page relative position after a rotation. The positions x y are
|
|
* relative to a page, i.e. 0=top of page, 1=bottom of page. They are NOT
|
|
* relative to the entire document.
|
|
*
|
|
* @param document the document
|
|
* @param x y the x y coordinates on the unrotated page
|
|
* @param xn yn the x y coordinates after rotation
|
|
*/
|
|
void page_calc_position(zathura_document_t* document, double x, double y,
|
|
double *xn, double *yn);
|
|
|
|
/**
|
|
* Converts a relative position within the document to a page number.
|
|
*
|
|
* @param document The document
|
|
* @param pos_x pos_y the position relative to the document
|
|
* @return page sitting in that position
|
|
*/
|
|
unsigned int position_to_page_number(zathura_document_t* document,
|
|
double pos_x, double pos_y);
|
|
|
|
/**
|
|
* Converts a page number to a position in units relative to the document
|
|
*
|
|
* We can specify where to aliwn the viewport and the page. For instance, xalign
|
|
* = 0 means align them on the left margin, xalign = 0.5 means centered, and
|
|
* xalign = 1.0 align them on the right margin.
|
|
*
|
|
* The return value is the position in in units relative to the document (0=top
|
|
* 1=bottom) of the point thet will lie at the center of the viewport.
|
|
*
|
|
* @param document The document
|
|
* @param page_number the given page number
|
|
* @param xalign yalign where to align the viewport and the page
|
|
* @return pos_x pos_y position that will lie at the center of the viewport.
|
|
*/
|
|
void page_number_to_position(zathura_document_t* document, unsigned int page_number,
|
|
double xalign, double yalign, double *pos_x, double *pos_y);
|
|
|
|
/**
|
|
* Checks whether a given page falls within the viewport
|
|
*
|
|
* @param document The document
|
|
* @param page_number the page number
|
|
* @return true if the page intersects the viewport
|
|
*/
|
|
bool page_is_visible(zathura_document_t *document, unsigned int page_number);
|
|
|
|
/**
|
|
* Clone a GtkAdjustment
|
|
*
|
|
* Creates a new adjustment with the same value, lower and upper bounds, step
|
|
* and page increments and page_size as the original adjustment.
|
|
*
|
|
* @param adjustment Adjustment instance to be cloned
|
|
* @return Pointer to the new adjustment
|
|
*/
|
|
GtkAdjustment* zathura_adjustment_clone(GtkAdjustment* adjustment);
|
|
|
|
/**
|
|
* Set the adjustment value while enforcing its limits
|
|
*
|
|
* @param adjustment Adjustment instance
|
|
* @param value Adjustment value
|
|
*/
|
|
void zathura_adjustment_set_value(GtkAdjustment* adjustment, gdouble value);
|
|
|
|
/**
|
|
* Compute the adjustment ratio
|
|
*
|
|
* That is, the ratio between the length from the lower bound to the middle of
|
|
* the slider, and the total length of the scrollbar.
|
|
*
|
|
* @param adjustment Scrollbar adjustment
|
|
* @return Adjustment ratio
|
|
*/
|
|
gdouble zathura_adjustment_get_ratio(GtkAdjustment* adjustment);
|
|
|
|
/**
|
|
* Set the adjustment value from ratio
|
|
*
|
|
* The ratio is usually obtained from a previous call to
|
|
* zathura_adjustment_get_ratio().
|
|
*
|
|
* @param adjustment Adjustment instance
|
|
* @param ratio Ratio from which the adjustment value will be set
|
|
*/
|
|
void zathura_adjustment_set_value_from_ratio(GtkAdjustment* adjustment,
|
|
gdouble ratio);
|
|
|
|
#endif /* ZATHURA_ADJUSTMENT_H */
|