mirror of
https://git.pwmt.org/pwmt/zathura.git
synced 2024-09-20 20:31:26 +02:00
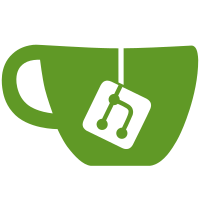
This patch adds some enhancements/fixes to the bookmarking feature of Zathura: - Bookmark the exact vertical and horizontal adjustments values, along with the page number. This is done in a backward-compatible way for both the plain and sqlite database backends, so that bookmarks that was taken previously (bookmarking only the page number) will still work as expected and won't be lost. - Fix the issue of not being able to remove bookmarks from the plain database; removing a bookmark in plain_remove_bookmark using g_key_file_remove_key corrupts the bookmarks file. This is due to not truncating the entire bookmarks file in zathura_db_write_key_file_to_file prior to overriding it with a new one not containing the removed line. This is why, I guess, plain_remove_bookmark hadn't been implemented as expected using g_key_file_remove_key, because apparently, someone thought that the problem is caused by this API. - Fix not being able to update existing bookmarks persistently; updating a bookmark works only during the current session, but after the file is closed and reopened, the updated bookmark still has it's old value. This is due to changing only the bookmark structure in memory; the proper way to do it, is to call zathura_db_remove_bookmark on the old bookmark, then follow that by a call to zathura_db_add_bookmark on a bookmark structure containing the new position and the same old ID. - Make zathura_bookmark_add updates the bookmark if it already exists, rather than doing this externally in cmd_bookmark_create. This allows us to have all the relevant girara_notify messages in cmd_bookmark_create. Signed-off-by: Sebastian Ramacher <sebastian+dev@ramacher.at>
67 lines
1.7 KiB
C
67 lines
1.7 KiB
C
/* See LICENSE file for license and copyright information */
|
|
|
|
#ifndef BOOKMARKS_H
|
|
#define BOOKMARKS_H
|
|
|
|
#include <stdbool.h>
|
|
#include "zathura.h"
|
|
|
|
struct zathura_bookmark_s
|
|
{
|
|
gchar* id;
|
|
unsigned int page;
|
|
double x;
|
|
double y;
|
|
};
|
|
|
|
typedef struct zathura_bookmark_s zathura_bookmark_t;
|
|
|
|
/**
|
|
* Create a bookmark and add it to the list of bookmarks.
|
|
* @param zathura The zathura instance.
|
|
* @param id The bookmark's id.
|
|
* @param page The bookmark's page.
|
|
* @return the bookmark instance or NULL on failure.
|
|
*/
|
|
zathura_bookmark_t* zathura_bookmark_add(zathura_t* zathura, const gchar* id, unsigned int page);
|
|
|
|
/**
|
|
* Remove a bookmark from the list of bookmarks.
|
|
* @param zathura The zathura instance.
|
|
* @param id The bookmark's id.
|
|
* @return true on success, false otherwise
|
|
*/
|
|
bool zathura_bookmark_remove(zathura_t* zathura, const gchar* id);
|
|
|
|
/**
|
|
* Get bookmark from the list of bookmarks.
|
|
* @param zathura The zathura instance.
|
|
* @param id The bookmark's id.
|
|
* @return The bookmark instance if it exists or NULL otherwise.
|
|
*/
|
|
zathura_bookmark_t* zathura_bookmark_get(zathura_t* zathura, const gchar* id);
|
|
|
|
/**
|
|
* Free a bookmark instance.
|
|
* @param bookmark The bookmark instance.
|
|
*/
|
|
void zathura_bookmark_free(zathura_bookmark_t* bookmark);
|
|
|
|
/**
|
|
* Load bookmarks for a specific file.
|
|
* @param zathura The zathura instance.
|
|
* @param file The file.
|
|
* @return true on success, false otherwise
|
|
*/
|
|
bool zathura_bookmarks_load(zathura_t* zathura, const gchar* file);
|
|
|
|
/**
|
|
* Compare two bookmarks.
|
|
* @param lhs a bookmark
|
|
* @param rhs a bookmark
|
|
* @returns g_strcmp0(lhs->id, rhs->id)
|
|
*/
|
|
int zathura_bookmarks_compare(zathura_bookmark_t* lhs, zathura_bookmark_t* rhs);
|
|
|
|
#endif // BOOKMARKS_H
|