mirror of
https://github.com/Jozufozu/Flywheel.git
synced 2025-01-11 14:56:12 +01:00
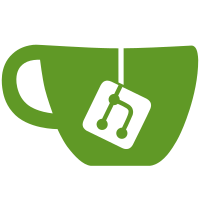
- WorldContext sort of uses a shader pipeline interface - Smarter import resolution - Reorganize shader sources - Rewritten shader templating - Still need builtin support
46 lines
995 B
Java
46 lines
995 B
Java
package com.jozufozu.flywheel.backend;
|
|
|
|
import java.util.ArrayList;
|
|
import java.util.List;
|
|
|
|
import javax.annotation.Nullable;
|
|
|
|
import com.jozufozu.flywheel.backend.pipeline.SourceFile;
|
|
import com.jozufozu.flywheel.backend.pipeline.error.ErrorReporter;
|
|
import com.jozufozu.flywheel.backend.pipeline.span.Span;
|
|
|
|
import net.minecraft.util.ResourceLocation;
|
|
|
|
public class FileResolution {
|
|
|
|
private final List<Span> foundSpans = new ArrayList<>();
|
|
private final ResourceLocation fileLoc;
|
|
private SourceFile file;
|
|
|
|
|
|
public FileResolution(ResourceLocation fileLoc) {
|
|
this.fileLoc = fileLoc;
|
|
}
|
|
|
|
public ResourceLocation getFileLoc() {
|
|
return fileLoc;
|
|
}
|
|
|
|
@Nullable
|
|
public SourceFile getFile() {
|
|
return file;
|
|
}
|
|
|
|
public void addSpan(Span span) {
|
|
foundSpans.add(span);
|
|
}
|
|
|
|
public void resolve(ShaderSources sources) {
|
|
|
|
try {
|
|
file = sources.source(fileLoc);
|
|
} catch (RuntimeException error) {
|
|
ErrorReporter.generateSpanError(foundSpans.get(0), "could not find source");
|
|
}
|
|
}
|
|
}
|